面向对象程序设计:编写程序,按照字典序列出一个文件中的所有不同单词及其出现次数。并给出main函数测试程序
时间: 2024-02-25 11:59:06 浏览: 123
好的,我可以为您提供一个使用C++语言实现的面向对象程序设计,以读取一个文件并按字典序列出所有不同的单词及其出现次数。下面是代码:
```cpp
#include <iostream>
#include <fstream>
#include <map>
#include <string>
#include <algorithm>
class WordCounter {
public:
WordCounter() {}
void countWords(const std::string& filename) {
std::ifstream inFile(filename);
if (!inFile) {
std::cerr << "Error opening file: " << filename << std::endl;
exit(1);
}
std::string word;
while (inFile >> word) {
// Convert word to lowercase
std::transform(word.begin(), word.end(), word.begin(), ::tolower);
// Remove non-alphabetic characters
word.erase(std::remove_if(word.begin(), word.end(), [](char c) {
return !std::isalpha(c);
}), word.end());
if (word.length() > 0) {
// Increment word count
wordCounts[word]++;
}
}
inFile.close();
}
void printWordCounts() const {
for (const auto& pair : wordCounts) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
}
private:
std::map<std::string, int> wordCounts;
};
int main() {
WordCounter wordCounter;
std::string filename = "example.txt";
wordCounter.countWords(filename);
wordCounter.printWordCounts();
return 0;
}
```
在这个程序中,我们定义了一个名为 `WordCounter` 的类,其中包含了两个公有函数:`countWords` 和 `printWordCounts`。`countWords` 函数用于读取指定的文件并计算每个不同单词的出现次数,将结果存储在一个 `std::map` 中。`printWordCounts` 函数则用于打印出每个不同单词及其出现次数。
在 `main` 函数中,我们首先创建了一个 `WordCounter` 对象 `wordCounter`,并指定了要读取的文件名。然后调用 `countWords` 函数计算出现次数,并最后调用 `printWordCounts` 函数打印结果。
请注意,我们在读取单词时先将其转换为小写,并删除所有非字母字符。这样可以确保在计算单词出现次数时不会因为大小写或标点符号的差异而产生重复计数。
希望这个例子能够帮助您理解面向对象程序设计的基本思想和实现方法。
阅读全文
相关推荐
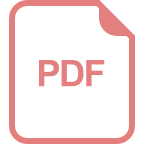
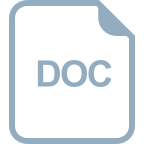
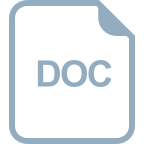
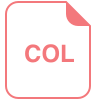
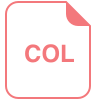
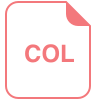
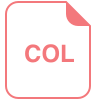
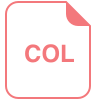
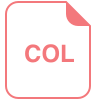
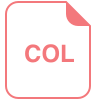
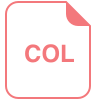




