二叉树已知中后序求二叉树
时间: 2023-11-14 14:14:42 浏览: 136
可以利用中序遍历和后序遍历序列构建出二叉树。具体步骤如下:
1. 后序遍历的最后一个元素即为根节点,将其创建为一个新的节点。
2. 在中序遍历中找到根节点的位置,该位置左侧的元素为左子树的中序遍历序列,右侧的元素为右子树的中序遍历序列。
3. 根据左子树的中序遍历序列长度,可以在后序遍历序列中确定左子树的后序遍历序列和右子树的后序遍历序列。
4. 递归处理左子树和右子树,重复上述步骤,分别创建左子树和右子树的根节点,然后将其挂载到当前节点下。
下面是一个示例代码实现:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def buildTree(inorder, postorder):
if not inorder or not postorder:
return None
root_val = postorder[-1]
root = TreeNode(root_val)
root_index = inorder.index(root_val)
left_inorder = inorder[:root_index]
right_inorder = inorder[root_index+1:]
left_postorder = postorder[:len(left_inorder)]
right_postorder = postorder[len(left_inorder):-1]
root.left = buildTree(left_inorder, left_postorder)
root.right = buildTree(right_inorder, right_postorder)
return root
```
其中,`inorder` 和 `postorder` 分别为中序遍历和后序遍历序列。可以通过调用 `buildTree` 函数来构建二叉树。
阅读全文
相关推荐





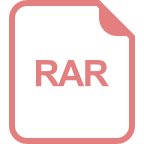
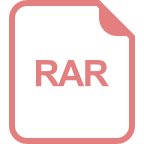
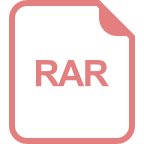


