基于SQLite3的通讯录管理系统
时间: 2023-12-07 18:05:09 浏览: 57
好的,基于SQLite3的通讯录管理系统可以实现以下功能:
1. 添加联系人:输入姓名、电话、地址等信息,添加到通讯录中。
2. 删除联系人:根据姓名或电话号码,删除指定的联系人。
3. 修改联系人:根据姓名或电话号码,修改指定联系人的信息。
4. 查询联系人:根据姓名或电话号码,查询指定联系人的信息。
5. 显示所有联系人:将通讯录中所有联系人的信息显示出来。
6. 导出联系人:将通讯录中所有联系人的信息导出到文件中。
7. 导入联系人:从文件中导入联系人信息到通讯录中。
8. 数据备份:将通讯录数据备份到文件中。
9. 数据恢复:从备份文件中恢复通讯录数据。
下面是一个基于Python的SQLite3通讯录管理系统的示例代码,你可以参考一下:
```python
import sqlite3
def create_table():
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS contacts
(name text, phone text, address text)''')
conn.commit()
conn.close()
def add_contact(name, phone, address):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("INSERT INTO contacts VALUES (?, ?, ?)", (name, phone, address))
conn.commit()
conn.close()
def delete_contact(name):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("DELETE FROM contacts WHERE name=?", (name,))
conn.commit()
conn.close()
def modify_contact(name, phone, address):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("UPDATE contacts SET phone=?, address=? WHERE name=?", (phone, address, name))
conn.commit()
conn.close()
def search_contact(name):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("SELECT * FROM contacts WHERE name=?", (name,))
result = c.fetchone()
conn.close()
return result
def show_all_contacts():
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("SELECT * FROM contacts")
results = c.fetchall()
conn.close()
return results
def export_contacts():
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("SELECT * FROM contacts")
results = c.fetchall()
with open('contacts.txt', 'w') as f:
for result in results:
f.write(','.join(result) + '\n')
conn.close()
def import_contacts():
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
with open('contacts.txt', 'r') as f:
for line in f:
line = line.strip()
if line:
name, phone, address = line.split(',')
c.execute("INSERT INTO contacts VALUES (?, ?, ?)", (name, phone, address))
conn.commit()
conn.close()
def backup_data():
conn = sqlite3.connect('contacts.db')
with open('contacts_backup.db', 'w') as f:
for line in conn.iterdump():
f.write('%s\n' % line)
conn.close()
def restore_data():
conn = sqlite3.connect('contacts.db')
with open('contacts_backup.db', 'r') as f:
sql_lines = f.read().splitlines()
for line in sql_lines:
conn.execute(line)
conn.commit()
conn.close()
create_table()
while True:
print('1. 添加联系人')
print('2. 删除联系人')
print('3. 修改联系人')
print('4. 查询联系人')
print('5. 显示所有联系人')
print('6. 导出联系人')
print('7. 导入联系人')
print('8. 数据备份')
print('9. 数据恢复')
print('0. 退出程序')
choice = input('请选择操作:')
if choice == '1':
name = input('请输入姓名:')
phone = input('请输入电话:')
address = input('请输入地址:')
add_contact(name, phone, address)
elif choice == '2':
name = input('请输入姓名:')
delete_contact(name)
elif choice == '3':
name = input('请输入姓名:')
phone = input('请输入电话:')
address = input('请输入地址:')
modify_contact(name, phone, address)
elif choice == '4':
name = input('请输入姓名:')
result = search_contact(name)
if result:
print(result)
else:
print('未找到联系人')
elif choice == '5':
results = show_all_contacts()
for result in results:
print(result)
elif choice == '6':
export_contacts()
print('导出成功')
elif choice == '7':
import_contacts()
print('导入成功')
elif choice == '8':
backup_data()
print('备份成功')
elif choice == '9':
restore_data()
print('恢复成功')
elif choice == '0':
print('再见!')
break
else:
print('无效选择,请重新输入')
```
相关推荐
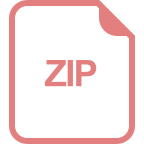
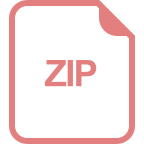













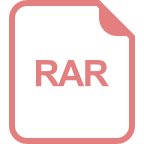
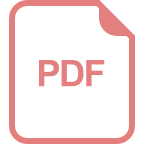