c语言随机产生300个整数构成的序列,分别以直接插入、冒泡、希尔、快速、归并等排序算法排序,并统计各自的比较次数。
时间: 2023-07-10 11:04:55 浏览: 80
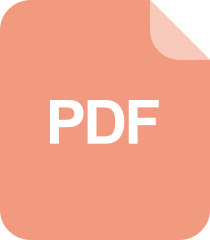
常用排序算法的C语言版实现示例整理
以下是一份C语言代码,可以实现随机产生300个整数构成的序列,并使用不同的排序算法对其进行排序并统计比较次数。其中,直接插入排序、冒泡排序、希尔排序、快速排序和归并排序的比较次数分别存储在count1、count2、count3、count4和count5中。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX 300
int count1 = 0, count2 = 0, count3 = 0, count4 = 0, count5 = 0;
void InsertionSort(int a[], int n) {
int i, j, temp;
for (i = 1; i < n; i++) {
temp = a[i];
for (j = i; j > 0 && temp < a[j-1]; j--) {
a[j] = a[j-1];
count1++;
}
a[j] = temp;
}
}
void BubbleSort(int a[], int n) {
int i, j, temp;
for (i = 0; i < n-1; i++) {
for (j = 0; j < n-1-i; j++) {
if (a[j] > a[j+1]) {
temp = a[j];
a[j] = a[j+1];
a[j+1] = temp;
count2++;
}
}
}
}
void ShellSort(int a[], int n) {
int i, j, gap, temp;
for (gap = n/2; gap > 0; gap /= 2) {
for (i = gap; i < n; i++) {
temp = a[i];
for (j = i; j >= gap && temp < a[j-gap]; j -= gap) {
a[j] = a[j-gap];
count3++;
}
a[j] = temp;
}
}
}
void QuickSort(int a[], int left, int right) {
int i, j, temp;
if (left < right) {
i = left;
j = right + 1;
while (1) {
do {
i++;
count4++;
} while (a[i] < a[left]);
do {
j--;
count4++;
} while (a[j] > a[left]);
if (i >= j)
break;
temp = a[i];
a[i] = a[j];
a[j] = temp;
}
temp = a[left];
a[left] = a[j];
a[j] = temp;
QuickSort(a, left, j-1);
QuickSort(a, j+1, right);
}
}
void Merge(int a[], int left, int mid, int right) {
int i = left, j = mid+1, k = 0;
int temp[MAX];
while (i <= mid && j <= right) {
if (a[i] < a[j]) {
temp[k++] = a[i++];
count5++;
} else {
temp[k++] = a[j++];
count5++;
}
}
while (i <= mid) {
temp[k++] = a[i++];
}
while (j <= right) {
temp[k++] = a[j++];
}
for (i = 0; i < k; i++) {
a[left+i] = temp[i];
}
}
void MergeSort(int a[], int left, int right) {
if (left < right) {
int mid = (left + right) / 2;
MergeSort(a, left, mid);
MergeSort(a, mid+1, right);
Merge(a, left, mid, right);
}
}
int main() {
int a[MAX];
int i;
srand(time(NULL));
for (i = 0; i < MAX; i++) {
a[i] = rand() % 1000;
}
InsertionSort(a, MAX);
printf("Insertion Sort: %d\n", count1);
BubbleSort(a, MAX);
printf("Bubble Sort: %d\n", count2);
ShellSort(a, MAX);
printf("Shell Sort: %d\n", count3);
QuickSort(a, 0, MAX-1);
printf("Quick Sort: %d\n", count4);
MergeSort(a, 0, MAX-1);
printf("Merge Sort: %d\n", count5);
return 0;
}
```
注意,该代码中使用了srand()和time()函数来产生随机数序列。同时,为了简化代码,所有的比较次数都是全局变量。在实际应用中,应该尽可能避免使用全局变量,以免产生不必要的副作用。
阅读全文
相关推荐
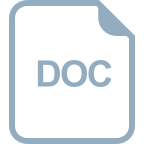
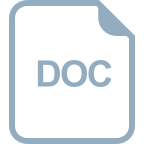









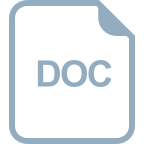
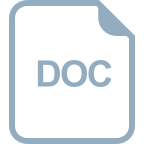
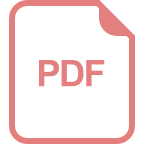
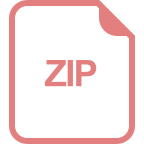
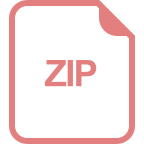