使用c语言关闭使用mkfifo创建的有名管道
时间: 2023-12-03 11:04:52 浏览: 141
关闭有名管道可以使用close函数,其参数为文件描述符,可以通过open函数获得。具体步骤如下:
1. 调用open函数打开有名管道文件,获得文件描述符。
2. 使用close函数关闭文件描述符。
示例代码如下:
```
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
char *fifo = "/tmp/myfifo"; // 有名管道文件名
// 打开有名管道文件
fd = open(fifo, O_WRONLY);
if (fd == -1) {
perror("open");
return -1;
}
// 写入数据 ...
write(fd, "Hello, world!", 13);
// 关闭文件描述符
close(fd);
return 0;
}
```
在上面的代码中,我们使用open函数打开有名管道文件,获得文件描述符fd,然后调用write函数向管道写入数据。最后使用close函数关闭文件描述符。
相关问题
在c语言重使用mkfifo创建有名管道并关闭
在 C 语言中,使用 `mkfifo` 函数可以创建一个有名管道。创建成功后,需要使用 `open` 函数打开管道,使用 `write` 函数写入数据或使用 `read` 函数读取数据。最后,使用 `close` 函数关闭管道。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
int main()
{
char *fifo = "/tmp/myfifo";
int fd;
mkfifo(fifo, 0666);
printf("FIFO created\n");
fd = open(fifo, O_WRONLY);
write(fd, "Hello, world!", 14);
close(fd);
printf("FIFO closed\n");
return 0;
}
```
在上面的代码中,首先使用 `mkfifo` 函数创建一个名为 `/tmp/myfifo` 的有名管道,并设置权限为 `0666`。然后,使用 `open` 函数打开管道,并使用 `write` 函数向管道中写入数据。最后,使用 `close` 函数关闭管道。
注意,在读写有名管道时,必须保证读写的进程都已经打开了管道。如果读写进程有任意一个进程没有打开管道,那么读写进程将会阻塞等待。
使用mkfifo函数创建有名管道,并基于该管道实现两个进程间的通信
在Unix或Linux系统中,使用`mkfifo`函数创建的是名为管道,即`named pipe`或`FIFO (First In First Out)`。它是半双工的,意味着只能在一个方向上传输数据。下面是一个简单的C语言示例,展示了如何创建一个有名管道并在两个进程中进行通信:
首先,我们需要创建管道文件:
```bash
mkdir -p /path/to/pipes
mkfifo /path/to/pipes/my_pipe
```
然后,在一个进程(例如`producer`)中:
```c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: producer <message>\n");
return 1;
}
const char *msg = argv[1];
int fd = open("/path/to/pipes/my_pipe", O_WRONLY); // 写模式打开
if (fd == -1) {
perror("Failed to open the named pipe");
return 1;
}
write(fd, msg, strlen(msg) + 1); // 发送消息到管道
close(fd);
printf("Producer sent message '%s'\n", msg);
return 0;
}
```
接着,在另一个进程(例如`consumer`)中:
```c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
if (argc != 1) {
printf("Usage: consumer\n");
return 1;
}
int fd = open("/path/to/pipes/my_pipe", O_RDONLY); // 读模式打开
if (fd == -1) {
perror("Failed to open the named pipe");
return 1;
}
char buffer[100]; // 预设接收缓冲区大小
read(fd, buffer, sizeof(buffer)); // 从管道接收数据
buffer[strlen(buffer) - 1] = '\0'; // 删除换行符
printf("Consumer received message '%s'\n", buffer);
close(fd);
return 0;
}
```
当你运行这两个程序时,一个进程(如`producer`)可以向`my_pipe`发送一条消息,另一个进程(如`consumer`)会接收到并显示出来。
阅读全文
相关推荐
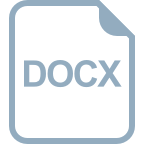
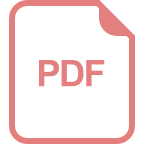
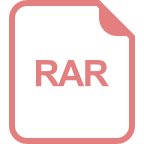

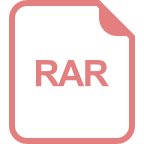
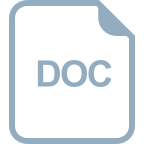
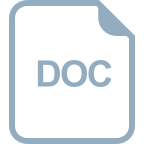
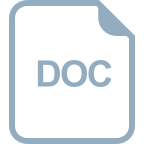
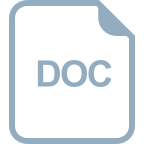
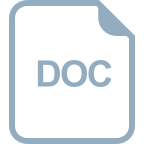
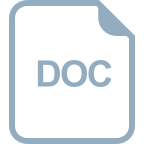
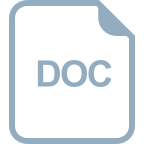
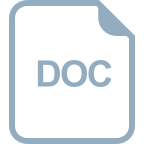
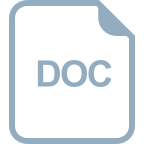
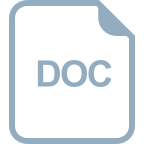

