result = os.system(order)如何输出到指定文件中
时间: 2024-02-28 12:57:35 浏览: 18
`os.system` 函数在执行系统命令时,不会将命令的输出信息保存下来,因此我们需要使用操作系统的重定向功能将命令的输出信息输出到指定文件中。具体方法如下:
```python
import os
# 打开文件,以追加方式写入
with open('output.txt', 'a') as f:
# 执行命令并将输出重定向到文件中
os.system('command > output.txt 2>&1')
```
在上面的代码中,我们使用了操作系统的重定向符号 `>` 将命令的标准输出重定向到文件 `output.txt` 中,`2>&1` 则表示将标准错误输出也重定向到标准输出。因此,所有的输出信息都会被写入到文件中。
注意,这种方法只能将命令的输出信息输出到文件中,无法获取命令的返回值。如果需要获取命令的返回值,可以使用 `subprocess` 模块中的 `check_output` 函数。
相关问题
def exec_shell(name ,cmd_str ,timeout=5 * 60): ret_values = [128] start_time = time.time() result = subprocess.Popen(cmd_str, shell=True) isrun = True while result.poll() is None and isrun: now = time.time() if now - start_time >= timeout and result.poll() is None: break time.sleep(1) ret = os.system('ssh -o ConnectTimeout=3 '+ name +' \"cd\"') if ret != 0: logging.info('%s Connect Timeout, ERROR: %d' % (name,ret)) isrun = False if result.poll() is None: result.terminate() # os.killpg(os.getpgid(result.pid), signal.SIGTERM) # os.kill(result.pid, signal.SIGKILL) kill_process(result.pid) ret_values[0] = 65535 else: ret_values[0] = result.returncode return ret_values[0]代码问题
这段代码看起来是用于执行 shell 命令的函数,但是其中有一些问题:
1. 在函数内部执行了一个无限循环,如果命令执行时间超过了指定的 timeout 时间,就会退出循环。但是这个循环会一直占用 CPU 资源,不太合理。可以使用 Python 的 `subprocess.call()` 函数来执行命令,并且可以通过 `timeout` 参数设置超时时间。
2. 在循环内部还执行了一个 `os.system()` 命令,这个命令会新开一个进程去执行 `ssh` 命令,这样会增加系统负担。可以使用 `paramiko` 库来实现 SSH 连接。
3. 函数最后返回的 `ret_values[0]` 只有一个元素,而且这个元素在函数内部已经被修改了,使用一个变量就可以了。
下面是修改后的代码:
```python
import subprocess
import time
import paramiko
def exec_shell(name, cmd_str, timeout=5*60):
start_time = time.time()
try:
result = subprocess.run(cmd_str, shell=True, check=True, timeout=timeout)
ret = result.returncode
except subprocess.TimeoutExpired:
ret = 65535
except subprocess.CalledProcessError as e:
ret = e.returncode
except Exception as e:
ret = 128
# 这里可以根据返回值做相应的处理,比如记录日志等
return ret
def ssh_connect(name):
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
ssh.connect(name, timeout=3)
return True
except:
return False
def exec_ssh_cmd(name, cmd_str, timeout=5*60):
start_time = time.time()
while True:
if ssh_connect(name):
break
now = time.time()
if now - start_time >= timeout:
logging.info('%s Connect Timeout' % name)
return 65535
time.sleep(1)
stdin, stdout, stderr = ssh.exec_command(cmd_str, timeout=timeout)
ret = stdout.channel.recv_exit_status()
# 这里可以根据返回值做相应的处理,比如记录日志等
return ret
```
其中 `exec_shell()` 函数使用了 Python 内置的 `subprocess.run()` 函数来执行 shell 命令,可以设置超时时间和检查命令是否执行成功的选项,这样就不需要使用循环了。
另外,我还添加了一个 `ssh_connect()` 函数来判断是否能够连接到远程服务器,以及一个 `exec_ssh_cmd()` 函数来执行 SSH 命令。这两个函数使用了 `paramiko` 库来实现 SSH 连接和命令执行,比较方便和安全。
path = os.path.join(os.getcwd(), 'result')
`os.path.join(os.getcwd(), 'result')`是一个用于生成文件路径的函数调用。`os.getcwd()`返回当前工作目录的路径,而`'result'`是要拼接到路径末尾的目录名。
这个函数的作用是将当前工作目录和目录名拼接在一起,生成一个完整的文件路径。具体来说,它会根据操作系统的不同,在路径中添加适当的分隔符(例如在Windows系统中是反斜杠`\`,在Unix系统中是正斜杠`/`),以确保生成的路径是正确的。
假设当前工作目录是`/home/user`,那么调用`os.path.join(os.getcwd(), 'result')`将返回`/home/user/result`这个路径。
相关推荐
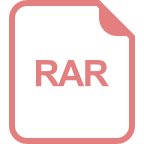
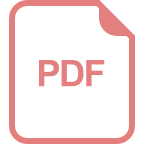
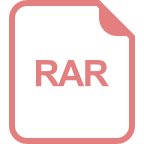













