could not broadcast input array from shape (72,1) into shape (72,)
时间: 2023-10-09 09:10:10 浏览: 290
这个错误通常是由于数组的形状不匹配导致的。在 NumPy 中,(72,1) 的形状表示一个二维数组,而 (72,) 的形状表示一个一维数组。当你尝试广播不同形状的数组时,就会出现这个错误。
解决这个问题的方法之一是使用 `numpy.squeeze()` 函数来移除数组中的单维度,从而将形状从 (72,1) 转换为 (72,)。例如:
```
import numpy as np
a = np.ones((72, 1))
b = np.arange(72)
c = a + b # 报错:could not broadcast input array from shape (72,1) into shape (72,)
a = np.squeeze(a)
c = a + b # 正常运行
```
另一种方法是使用 `numpy.reshape()` 函数来显式地调整数组的形状。例如:
```
import numpy as np
a = np.ones((72, 1))
b = np.arange(72)
c = a.reshape(72) + b # 正常运行
```
这两种方法都可以解决这个问题,具体使用哪一种取决于你的实际情况。
相关问题
could not broadcast input array from shape (12) into shape (16)
This error message indicates that you are trying to perform a broadcasting operation on two NumPy arrays that have incompatible shapes. Specifically, you are trying to broadcast an array with 12 elements into an array with 16 elements, which is not possible.
To fix this error, you need to ensure that the shapes of the arrays are compatible for broadcasting. One way to do this is to reshape the array with 12 elements to have the same shape as the array with 16 elements, or vice versa.
For example, if you have an array with shape (12,) and you want to broadcast it with an array with shape (4, 4), you can reshape the first array to have shape (4, 3) using the `reshape` method:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
b = np.zeros((4, 4))
a_reshaped = a.reshape((4, 3))
result = a_reshaped + b
```
Alternatively, you could reshape the second array to have shape (12,) using the `flatten` method:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
b = np.zeros((4, 4))
b_flat = b.flatten()
result = a + b_flat
```
Both of these approaches will ensure that the arrays have compatible shapes for broadcasting.
could not broadcast input array from shape (2) into shape (1,35)
This error message indicates that there is a mismatch between the dimensions of two arrays that you are trying to merge or broadcast together. Specifically, you are trying to broadcast an array with shape (2) onto an array with shape (1, 35), which is not possible.
To resolve this error, you will need to reshape or resize one or both of the arrays so that they have compatible shapes. One approach might be to reshape the (2) array to have shape (1, 2) using the np.reshape() function, and then broadcast it onto the (1, 35) array.
For example:
```
import numpy as np
a = np.array([1, 2])
b = np.zeros((1, 35))
a_reshaped = np.reshape(a, (1, 2))
b[:a_reshaped.shape[0], :a_reshaped.shape[1]] += a_reshaped
print(b)
```
This code reshapes the (2) array to have shape (1, 2), and then adds it to the (1, 35) array using array indexing. The resulting output should be a (1, 35) array with the first two elements set to the values of the (2) array.
阅读全文
相关推荐
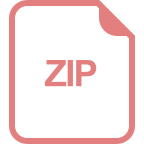
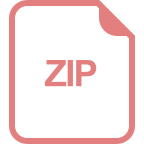
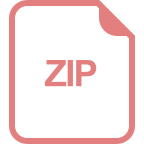













