could not broadcast input array from shape (100,) into shape (1000,)
时间: 2024-05-13 18:17:52 浏览: 157
这个错误通常发生在尝试将一维数组广播到另一个一维数组时,两个数组的长度不匹配。例如,将长度为100的一维数组广播到长度为1000的一维数组时,就会出现这个错误。
要解决这个问题,你需要确保两个数组的长度匹配,或者使用reshape()函数将一个数组转换为与另一个数组相同的形状。你也可以使用np.newaxis来添加维度,使得两个数组的形状相同。
以下是一个示例代码:
``` python
import numpy as np
# 创建一个长度为100的一维数组
a = np.zeros(100)
# 创建一个长度为1000的一维数组
b = np.zeros(1000)
# 将a数组重塑为长度为1000的一维数组
a = np.reshape(a, (1000,))
# 或者使用np.newaxis来添加维度
a = a[np.newaxis, :]
# 确保两个数组的长度相同
assert a.shape == b.shape
# 进行广播操作
c = a + b
```
这个示例代码中,我们先创建一个长度为100的一维数组`a`和一个长度为1000的一维数组`b`,然后使用`reshape()`函数将`a`数组转换为与`b`数组相同的形状。或者使用`np.newaxis`来添加一个新的维度,使得`a`数组的形状为`(1, 1000)`。最后使用`assert`语句来确保两个数组的形状相同,然后进行广播操作。
相关问题
could not broadcast input array from shape (12) into shape (16)
This error message indicates that you are trying to perform a broadcasting operation on two NumPy arrays that have incompatible shapes. Specifically, you are trying to broadcast an array with 12 elements into an array with 16 elements, which is not possible.
To fix this error, you need to ensure that the shapes of the arrays are compatible for broadcasting. One way to do this is to reshape the array with 12 elements to have the same shape as the array with 16 elements, or vice versa.
For example, if you have an array with shape (12,) and you want to broadcast it with an array with shape (4, 4), you can reshape the first array to have shape (4, 3) using the `reshape` method:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
b = np.zeros((4, 4))
a_reshaped = a.reshape((4, 3))
result = a_reshaped + b
```
Alternatively, you could reshape the second array to have shape (12,) using the `flatten` method:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
b = np.zeros((4, 4))
b_flat = b.flatten()
result = a + b_flat
```
Both of these approaches will ensure that the arrays have compatible shapes for broadcasting.
could not broadcast input array from shape (100,100,3) into shape (100,100)什么意思
这个错误信息意味着你在尝试将形状为 (100, 100, 3) 的数组广播到形状为 (100, 100) 的数组上,但两者的形状不兼容。广播是一种 NumPy 数组操作,它可以自动将一个形状较小的数组扩展到一个形状较大的数组,以便它们可以进行元素级别的运算。但是,广播有一些限制,例如两个数组的形状必须在某些维度上匹配,或者其中一个数组的形状在某些维度上必须为 1。如果这些限制没有被满足,就会出现这个错误。你需要检查你的代码,确保你正在进行正确的数组操作。
阅读全文
相关推荐
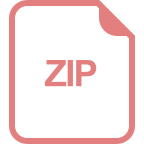
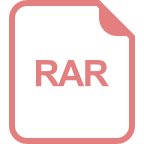
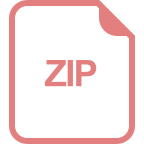













