could not broadcast input array from shape (0,) into shape (25,)
时间: 2024-11-03 11:23:36 浏览: 8
当遇到 "could not broadcast input array from shape (0,) into shape (25,)" 这样的错误时,这通常发生在尝试将一个尺寸为 `(0,)` 的数组(即长度为0的一维数组,也就是空数组)与一个预期为 `(25,)` 形状的数组进行操作,比如拼接、相加或 broadcasting(广播运算)的时候。在NumPy中,两个数组进行操作需要它们在维度上兼容,即至少有一个维度是相同的,并且当维度不同时,较小的那个维度需要能够通过复制自身填充较大的那个维度。
例如,如果你试图这样做:
```python
arr1 = np.empty(0) # 一个长度为0的数组
arr2 = np.array([1, 2, 3, ..., 24, 25]) # 长度为25的数组
# 这里会导致错误,因为 arr1.shape 是 (0,), 而 arr2.shape 是 (25,)
arr1 + arr2
```
在这种情况下,由于 arr1 没有任何元素,它无法按照所需的形状 (25,) 进行广播。解决这种问题的方式通常是确保你的输入数组至少有一个非零元素,或者明确地指定你要进行的操作的维度。
相关问题
could not broadcast input array from shape (12) into shape (16)
This error message indicates that you are trying to perform a broadcasting operation on two NumPy arrays that have incompatible shapes. Specifically, you are trying to broadcast an array with 12 elements into an array with 16 elements, which is not possible.
To fix this error, you need to ensure that the shapes of the arrays are compatible for broadcasting. One way to do this is to reshape the array with 12 elements to have the same shape as the array with 16 elements, or vice versa.
For example, if you have an array with shape (12,) and you want to broadcast it with an array with shape (4, 4), you can reshape the first array to have shape (4, 3) using the `reshape` method:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
b = np.zeros((4, 4))
a_reshaped = a.reshape((4, 3))
result = a_reshaped + b
```
Alternatively, you could reshape the second array to have shape (12,) using the `flatten` method:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
b = np.zeros((4, 4))
b_flat = b.flatten()
result = a + b_flat
```
Both of these approaches will ensure that the arrays have compatible shapes for broadcasting.
ValueError: could not broadcast input array from shape (0,) into shape (0,604800)
这个错误通常发生在尝试将一个空数组广播到一个非空数组的形状时。在你的情况下,你正在尝试将一个形状为(0,)的空数组广播到形状为(0, 604800)的非空数组。
这个错误可能是由于以下原因之一引起的:
1. 输入数组为空:请确保你的输入数组不是空数组。如果输入数组是空的,你需要确保在广播之前有正确的数据。
2. 形状不匹配:请检查你的输入数组和目标数组的形状是否匹配。在这种情况下,目标数组的形状是(0, 604800),这意味着它有0行和604800列。确保你的输入数组具有相同的列数,或者调整输入数组的形状以与目标数组兼容。
3. 数据类型错误:请确保你的输入数组具有与目标数组相同的数据类型。如果数据类型不匹配,可能无法进行广播操作。
请仔细检查你的代码并根据上述提示进行调整,以解决这个问题。如有需要,请提供更多关于你的代码和数据的详细信息,以便我们能够给出更具体的建议。
阅读全文
相关推荐
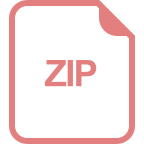
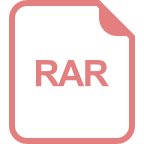
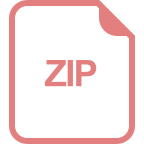













