java怎样将一个承载文件路径的List<String> pathList变成List<File>fileList
时间: 2023-08-16 20:03:25 浏览: 70
可以使用Java 8的流(Stream)来将List<String>转换为List<File>,具体代码如下:
```java
List<File> fileList = pathList.stream()
.map(File::new)
.collect(Collectors.toList());
```
上述代码中,使用map方法将String类型的路径转换为File类型,然后使用collect方法将转换后的File对象收集到List中。
相关问题
对于一个List<String> pathList,怎样编写代码删除其第一个字符串
可以使用 `remove()` 方法来删除 `List` 中的元素,如下所示:
```java
List<String> pathList = new ArrayList<>();
// 假设 pathList 至少包含一个元素
pathList.remove(0); // 删除第一个元素
```
`remove()` 方法接受一个 `int` 类型的参数,表示要删除的元素的索引。在上面的代码中,我们将索引设为 0,表示删除第一个元素。执行完上述代码后,`pathList` 中的第一个元素就被删除了。
将Android一个HashMap<String, List<String>>中的value值代表文件路径,怎样将value值代表的文件名和文件路径分别赋值给Item有两行字的ListView了。
您可以使用以下代码将HashMap中的value值(文件路径)转换为文件名和路径,并将其添加到ListView中:
```java
HashMap<String, List<String>> hashMap = new HashMap<String, List<String>>(); // 假设 HashMap 已经有值
List<String> fileList = new ArrayList<String>();
for (List<String> pathList : hashMap.values()) {
fileList.addAll(pathList);
}
List<String> fileNames = new ArrayList<String>();
for (String path : fileList) {
File file = new File(path);
fileNames.add(file.getName()); // 获取文件名
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_2, android.R.id.text1, fileNames) {
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = super.getView(position, convertView, parent);
TextView text1 = (TextView) view.findViewById(android.R.id.text1);
TextView text2 = (TextView) view.findViewById(android.R.id.text2);
File file = new File(fileList.get(position));
text1.setText(file.getName()); // 设置文件名
text2.setText(file.getPath()); // 设置文件路径
return view;
}
};
```
这个代码中,我们先将HashMap中的value值(文件路径)全部提取出来,然后逐个获取文件名,最终将文件名和文件路径分别设置到ListView的两行文本中。注意,在ListView中显示两行文本需要使用自定义的ArrayAdapter,并在getView()方法中设置两行文本的内容。
相关推荐
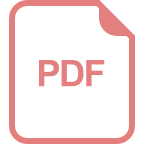
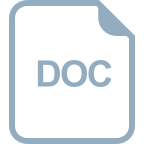












