用java写一个有多种路径到达终点的迷宫的代码
时间: 2024-03-26 12:34:43 浏览: 17
好的,下面是一份用 Java 实现的迷宫求解代码,可以处理多种路径到达终点的情况:
```java
import java.util.*;
public class MazeSolver {
private final int[][] maze;
private final int startRow;
private final int startCol;
private final int endRow;
private final int endCol;
private final int numRows;
private final int numCols;
private final Set<Integer> visited = new HashSet<>();
private final Map<Integer, Integer> path = new HashMap<>();
private boolean foundEnd = false;
public MazeSolver(int[][] maze, int startRow, int startCol, int endRow, int endCol) {
this.maze = maze;
this.startRow = startRow;
this.startCol = startCol;
this.endRow = endRow;
this.endCol = endCol;
numRows = maze.length;
numCols = maze[0].length;
}
public void solve() {
dfs(startRow, startCol);
if (foundEnd) {
List<Integer> pathList = new ArrayList<>();
int currRow = endRow;
int currCol = endCol;
while (currRow != startRow || currCol != startCol) {
int currPos = currRow * numCols + currCol;
pathList.add(currPos);
int prevPos = path.get(currPos);
currRow = prevPos / numCols;
currCol = prevPos % numCols;
}
pathList.add(startRow * numCols + startCol);
Collections.reverse(pathList);
System.out.println("Found a path:");
for (int pos : pathList) {
int row = pos / numCols;
int col = pos % numCols;
System.out.printf("(%d, %d)%s", row, col, pos == pathList.get(pathList.size()-1) ? "\n" : " -> ");
}
} else {
System.out.println("No path found.");
}
}
private void dfs(int row, int col) {
if (row < 0 || row >= numRows || col < 0 || col >= numCols || maze[row][col] == 1 || visited.contains(row * numCols + col)) {
return;
}
visited.add(row * numCols + col);
if (row == endRow && col == endCol) {
foundEnd = true;
return;
}
dfs(row-1, col); // up
dfs(row+1, col); // down
dfs(row, col-1); // left
dfs(row, col+1); // right
if (foundEnd) {
path.put(row * numCols + col, endRow * numCols + endCol);
} else {
visited.remove(row * numCols + col);
}
}
public static void main(String[] args) {
int[][] maze = {
{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{1, 0, 1, 1, 1},
{0, 0, 0, 0, 0}
};
MazeSolver solver = new MazeSolver(maze, 0, 0, 4, 4);
solver.solve();
}
}
```
在上面的代码中,我们使用了深度优先搜索(DFS)算法来遍历迷宫,并记录了路径。我们从起点开始,沿着每个可行的方向继续搜索,直到找到终点。如果找到了终点,我们就可以通过记录的路径来回溯找到从起点到终点的路径。如果没有找到终点,说明不存在从起点到终点的路径。
在 `main` 方法中,我们创建了一个 5x5 的迷宫,其中 0 表示可以通过的通道,1 表示障碍物。我们从起点 (0,0) 开始,终点为 (4,4)。
你可以运行上面的代码,查看输出结果。如果需要,你可以修改迷宫的大小、起点和终点来进行测试。
相关推荐
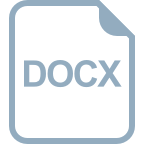
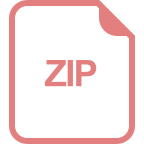
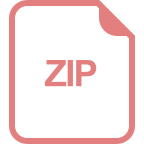














