用javafx写一个迷宫游戏要求有自动寻路功能
时间: 2023-11-27 22:55:32 浏览: 129
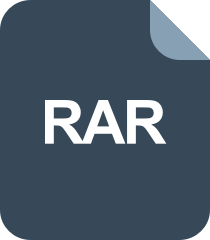
基于JAVA的迷宫自动寻路算法实现

好的,以下是一个简单的迷宫游戏实现方案,其中包括自动寻路功能。需要注意的是,这只是一个初步的示例程序,实际开发中可能需要进行更多的优化和改进。
首先,我们需要设计迷宫地图的数据结构。我们可以用一个二维数组来表示迷宫,其中0表示通路,1表示墙壁。例如,下面的数组表示一个5x5的迷宫地图:
```
int[][] maze = {
{1, 1, 1, 1, 1},
{1, 0, 0, 0, 1},
{1, 0, 1, 0, 1},
{1, 0, 0, 0, 1},
{1, 1, 1, 1, 1}
};
```
然后,我们可以用JavaFX来实现迷宫游戏的界面。我们可以使用一个GridPane来展示迷宫地图。
```
GridPane gridPane = new GridPane();
for (int i = 0; i < maze.length; i++) {
for (int j = 0; j < maze[0].length; j++) {
Rectangle rect = new Rectangle(50, 50);
if (maze[i][j] == 1) {
rect.setFill(Color.BLACK);
} else {
rect.setFill(Color.WHITE);
}
gridPane.add(rect, j, i);
}
}
```
接着,我们需要实现自动寻路算法。这里我们可以使用广度优先搜索算法(BFS)来寻找从起点到终点的最短路径。具体实现如下:
```
int[][] dirs = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}}; // 上下左右四个方向
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startRow, startCol}); // 将起点加入队列
while (!queue.isEmpty()) {
int[] curr = queue.poll();
int row = curr[0];
int col = curr[1];
if (row == endRow && col == endCol) { // 找到终点,退出循环
break;
}
for (int[] dir : dirs) {
int newRow = row + dir[0];
int newCol = col + dir[1];
if (newRow >= 0 && newRow < numRows && newCol >= 0 && newCol < numCols && maze[newRow][newCol] == 0 && !visited[newRow][newCol]) {
queue.offer(new int[]{newRow, newCol}); // 将相邻的通路加入队列
visited[newRow][newCol] = true; // 标记为已访问
prev[newRow][newCol] = curr; // 记录前一个位置
}
}
}
```
最后,我们可以使用一个Stack来记录从终点到起点的路径,并在界面上展示出来。
```
Stack<int[]> stack = new Stack<>();
int[] curr = new int[]{endRow, endCol};
while (curr != null) {
stack.push(curr);
curr = prev[curr[0]][curr[1]];
}
while (!stack.isEmpty()) {
int[] pos = stack.pop();
Rectangle rect = new Rectangle(50, 50, Color.GREEN);
gridPane.add(rect, pos[1], pos[0]);
}
```
完整的代码如下:
```
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.GridPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import java.util.LinkedList;
import java.util.Queue;
import java.util.Stack;
public class MazeGame extends Application {
private int[][] maze = {
{1, 1, 1, 1, 1},
{1, 0, 0, 0, 1},
{1, 0, 1, 0, 1},
{1, 0, 0, 0, 1},
{1, 1, 1, 1, 1}
};
private int numRows = maze.length;
private int numCols = maze[0].length;
private boolean[][] visited = new boolean[numRows][numCols];
private int[][] prev = new int[numRows][numCols];
private int startRow = 1;
private int startCol = 1;
private int endRow = 3;
private int endCol = 3;
@Override
public void start(Stage primaryStage) throws Exception {
GridPane gridPane = new GridPane();
for (int i = 0; i < maze.length; i++) {
for (int j = 0; j < maze[0].length; j++) {
Rectangle rect = new Rectangle(50, 50);
if (maze[i][j] == 1) {
rect.setFill(Color.BLACK);
} else {
rect.setFill(Color.WHITE);
}
gridPane.add(rect, j, i);
}
}
int[][] dirs = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startRow, startCol});
while (!queue.isEmpty()) {
int[] curr = queue.poll();
int row = curr[0];
int col = curr[1];
if (row == endRow && col == endCol) {
break;
}
for (int[] dir : dirs) {
int newRow = row + dir[0];
int newCol = col + dir[1];
if (newRow >= 0 && newRow < numRows && newCol >= 0 && newCol < numCols && maze[newRow][newCol] == 0 && !visited[newRow][newCol]) {
queue.offer(new int[]{newRow, newCol});
visited[newRow][newCol] = true;
prev[newRow][newCol] = curr;
}
}
}
Stack<int[]> stack = new Stack<>();
int[] curr = new int[]{endRow, endCol};
while (curr != null) {
stack.push(curr);
curr = prev[curr[0]][curr[1]];
}
while (!stack.isEmpty()) {
int[] pos = stack.pop();
Rectangle rect = new Rectangle(50, 50, Color.GREEN);
gridPane.add(rect, pos[1], pos[0]);
}
Scene scene = new Scene(gridPane);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
阅读全文
相关推荐

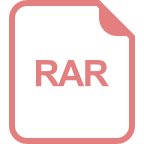
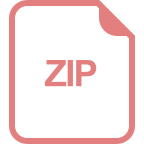
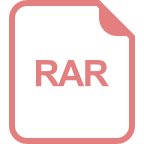
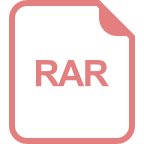
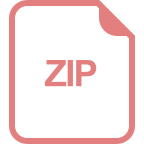
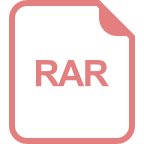
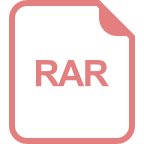
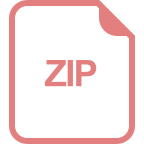
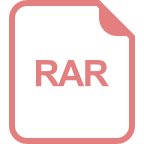
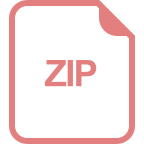
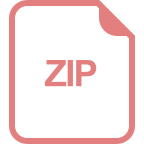
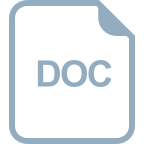

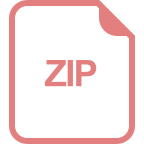