用java写一个迷宫游戏代码
时间: 2024-04-30 13:18:44 浏览: 102
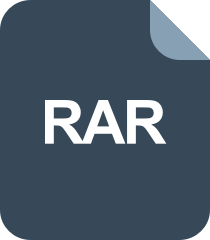
JAVA 实现《复杂迷宫》游戏-全部源码

以下是一个简单的迷宫游戏代码示例,使用 Java 编写。它使用二维数组来表示迷宫,其中 0 表示空路径,1 表示墙壁,2 表示终点。玩家可以使用方向键来移动,尝试从起点到达终点。
```java
import java.util.Scanner;
public class MazeGame {
public static void main(String[] args) {
int[][] maze = {
{1, 1, 1, 1, 1, 1, 1, 1, 1, 1},
{1, 0, 0, 1, 0, 0, 0, 1, 0, 1},
{1, 0, 0, 1, 0, 0, 0, 1, 0, 1},
{1, 0, 0, 0, 0, 1, 1, 0, 0, 1},
{1, 0, 1, 1, 1, 0, 0, 0, 0, 1},
{1, 0, 0, 0, 1, 0, 0, 0, 0, 1},
{1, 0, 1, 0, 0, 0, 1, 0, 0, 1},
{1, 0, 1, 1, 1, 0, 1, 1, 0, 1},
{1, 1, 0, 0, 0, 0, 0, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 1, 1, 1, 1}
};
int startX = 1;
int startY = 1;
int endX = 8;
int endY = 8;
int currentX = startX;
int currentY = startY;
Scanner scanner = new Scanner(System.in);
while (true) {
// 打印迷宫
for (int i = 0; i < maze.length; i++) {
for (int j = 0; j < maze[i].length; j++) {
if (i == currentY && j == currentX) {
System.out.print("P ");
} else if (maze[i][j] == 0) {
System.out.print(" ");
} else if (maze[i][j] == 1) {
System.out.print("█ ");
} else if (maze[i][j] == 2) {
System.out.print("E ");
}
}
System.out.println();
}
// 判断是否到达终点
if (currentX == endX && currentY == endY) {
System.out.println("恭喜你,你赢了!");
break;
}
// 获取玩家输入
System.out.print("请输入方向(上:w,下:s,左:a,右:d):");
String input = scanner.nextLine().toLowerCase();
// 移动玩家
switch (input) {
case "w":
if (maze[currentY - 1][currentX] != 1) {
currentY--;
}
break;
case "s":
if (maze[currentY + 1][currentX] != 1) {
currentY++;
}
break;
case "a":
if (maze[currentY][currentX - 1] != 1) {
currentX--;
}
break;
case "d":
if (maze[currentY][currentX + 1] != 1) {
currentX++;
}
break;
default:
System.out.println("无效输入!");
break;
}
}
}
}
```
这只是一个简单的示例代码,你可以根据自己的需求和喜好进行修改和扩展,例如添加更多关卡、改进迷宫生成算法、增加障碍物等等。
阅读全文
相关推荐
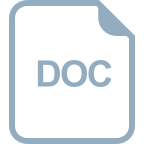
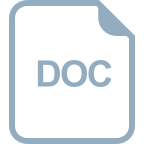



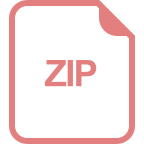
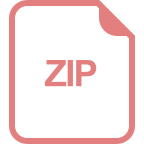
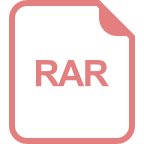
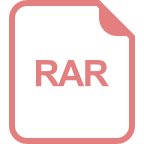







