java实现走迷宫游戏代码
时间: 2023-11-12 20:01:06 浏览: 184
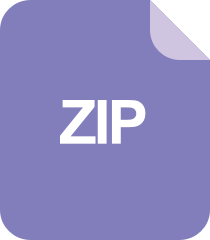
java小游戏——走迷宫 走马.zip
你好,以下是 Java 实现走迷宫游戏的代码:
```java
import java.util.*;
public class MazeSolver {
private int[][] maze; // 迷宫
private int[] start; // 起点坐标
private int[] end; // 终点坐标
private Map<String, int[]> directions; // 方向集合
public MazeSolver(int[][] maze, int[] start, int[] end) {
this.maze = maze;
this.start = start;
this.end = end;
this.directions = new HashMap<>();
directions.put("up", new int[]{-1, 0});
directions.put("down", new int[]{1, 0});
directions.put("left", new int[]{0, -1});
directions.put("right", new int[]{0, 1});
}
public List<int[]> solve() {
List<int[]> path = new ArrayList<>();
Set<String> visited = new HashSet<>();
Stack<int[]> stack = new Stack<>();
stack.push(start);
while (!stack.isEmpty()) {
int[] current = stack.pop();
path.add(current);
if (Arrays.equals(current, end)) {
return path;
}
for (Map.Entry<String, int[]> entry : directions.entrySet()) {
int[] direction = entry.getValue();
int[] next = new int[]{current[0] + direction[0], current[1] + direction[1]};
String key = next[0] + "," + next[1];
if (next[0] >= 0 && next[0] < maze.length
&& next[1] >= 0 && next[1] < maze[0].length
&& maze[next[0]][next[1]] == 0
&& !visited.contains(key)) {
stack.push(next);
visited.add(key);
}
}
}
return null;
}
public static void main(String[] args) {
int[][] maze = new int[][]{
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 1, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 0, 0}
};
int[] start = new int[]{1, 1};
int[] end = new int[]{3, 3};
MazeSolver solver = new MazeSolver(maze, start, end);
List<int[]> path = solver.solve();
if (path != null) {
System.out.println("Path: ");
for (int[] point : path) {
System.out.println(Arrays.toString(point));
}
} else {
System.out.println("No path found.");
}
}
}
```
希望能对您有所帮助。如果您有其他问题,可以直接问我哦~
阅读全文
相关推荐
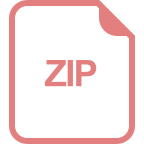
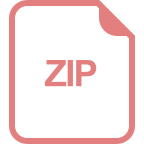















