int main() { int i; char *p; char *p1="gec123456"; printf("Please input a password:\n"); scanf("%s",p); for(i=0;i<2;i++) { if(strcmp(p,p1)==0) { printf("%ld",strlen(p)); break; } else { printf("Password error,please enter the password again\n"); scanf("%s",p); } } printf("\n"); } 代码纠错
时间: 2024-01-07 18:03:09 浏览: 26
该代码存在几个问题:
1. 定义了一个字符指针变量 `p`,但没有分配内存空间,直接使用会导致未知的行为。
2. 在使用 `strcmp()` 函数比较字符串时,需要先分配空间并将输入的字符串复制到该空间中,然后再与 `p1` 进行比较。
3. 在循环中,只允许用户输入两次密码,但循环条件只判断了 `i<2`,而没有判断密码是否正确。如果密码错误,循环将会无限进行下去。
下面是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 20
int main() {
int i;
char password[MAX_LEN]; // 定义一个字符数组来存储密码
char *p1 = "gec123456";
printf("Please input a password:\n");
scanf("%s", password);
for (i = 0; i < 2 && strcmp(password, p1) != 0; i++) {
printf("Password error, please enter the password again\n");
scanf("%s", password);
}
if (strcmp(password, p1) == 0) {
printf("The length of the password is %ld\n", strlen(password));
} else {
printf("You have entered the wrong password too many times.\n");
}
return 0;
}
```
在修改后的代码中,我们定义了一个字符数组 `password` 来存储用户输入的密码,长度为 `MAX_LEN`。在循环中,通过 `strcmp()` 函数比较密码是否正确,如果不正确,提示用户重新输入。循环条件中增加了 `strcmp()` 的判断,如果密码正确则退出循环,否则最多允许用户输入两次密码。在循环结束后,再根据密码是否正确输出相应的提示信息。
相关推荐
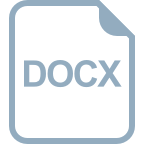
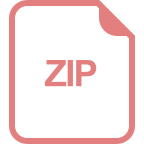














