mercury=(190,190,190),np = Neopixel(pin,LED_NUM),np[0]=mercury,np.write 这几行代码里np[0]=mercury这行代码啥意思
时间: 2024-04-20 12:26:50 浏览: 114
在这段代码中,"np[0]=mercury"这行代码的意思是将名为"mercury"的颜色值赋给了Neopixel对象np中的第一个LED灯。Neopixel是一个用于控制彩色LED灯带的库,其中np[0]表示第一个LED灯的索引,而"mercury=(190,190,190)"表示一种颜色的RGB值,即红色、绿色和蓝色通道的亮度值。通过将颜色值赋给特定的LED灯,可以实现对LED灯的控制和显示特定的颜色。最后,"np.write"是将所做的更改应用到实际硬件上,使得LED灯显示出相应的颜色。
相关问题
class Question: def __init__(self, stem, options, answer): self.stem = stem self.options = options self.answer = answerclass QuestionBank: def __init__(self): self.questions = [] def add_question(self, question): self.questions.append(question) def remove_question(self, question): self.questions.remove(question) def get_random_questions(self, num): return random.sample(self.questions, num)class Paper: def __init__(self, questions): self.questions = questions self.answers = {} def answer_question(self, question, answer): self.answers[question] = answer def get_score(self): score = 0 for question, answer in self.answers.items(): if answer == question.answer: score += 1 return scoreclass Grader: def __init__(self, paper): self.paper = paper def grade(self): return self.paper.get_score()# Example usagequestion1 = Question("What is the capital of France?", ["Paris", "London", "Berlin", "Madrid"], "Paris")question2 = Question("What is the largest planet in the solar system?", ["Mercury", "Venus", "Earth", "Jupiter"], "Jupiter")question3 = Question("What is the highest mountain in the world?", ["K2", "Mount Everest", "Makalu", "Cho Oyu"], "Mount Everest")question_bank = QuestionBank()question_bank.add_question(question1)question_bank.add_question(question2)question_bank.add_question(question3)paper = Paper(question_bank.get_random_questions(2))paper.answer_question(question1, "Paris")paper.answer_question(question2, "Jupiter")grader = Grader(paper)score = grader.grade()print("Your score is:", score)将这个代码转为C++的
好的,以下是将 Python 代码转换为 C++ 代码的示例:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <string>
#include <algorithm>
#include <random>
using namespace std;
class Question {
public:
string stem;
vector<string> options;
string answer;
Question(string s, vector<string> o, string a) {
stem = s;
options = o;
answer = a;
}
};
class QuestionBank {
public:
vector<Question> questions;
void add_question(Question q) {
questions.push_back(q);
}
void remove_question(Question q) {
questions.erase(remove(questions.begin(), questions.end(), q), questions.end());
}
vector<Question> get_random_questions(int num) {
vector<Question> res;
random_device rd;
mt19937 gen(rd());
shuffle(questions.begin(), questions.end(), gen);
for (int i = 0; i < num; ++i) {
res.push_back(questions[i]);
}
return res;
}
};
class Paper {
public:
vector<Question> questions;
map<Question, string> answers;
Paper(vector<Question> q) {
questions = q;
}
void answer_question(Question q, string a) {
answers[q] = a;
}
int get_score() {
int score = 0;
for (auto const& x : answers) {
if (x.second == x.first.answer) {
score++;
}
}
return score;
}
};
class Grader {
public:
Paper paper;
Grader(Paper p) {
paper = p;
}
int grade() {
return paper.get_score();
}
};
int main() {
Question question1("What is the capital of France?", {"Paris", "London", "Berlin", "Madrid"}, "Paris");
Question question2("What is the largest planet in the solar system?", {"Mercury", "Venus", "Earth", "Jupiter"}, "Jupiter");
Question question3("What is the highest mountain in the world?", {"K2", "Mount Everest", "Makalu", "Cho Oyu"}, "Mount Everest");
QuestionBank question_bank;
question_bank.add_question(question1);
question_bank.add_question(question2);
question_bank.add_question(question3);
vector<Question> random_questions = question_bank.get_random_questions(2);
Paper paper(random_questions);
paper.answer_question(question1, "Paris");
paper.answer_question(question2, "Jupiter");
Grader grader(paper);
int score = grader.grade();
cout << "Your score is: " << score << endl;
return 0;
}
```
注意,这只是一个简单的示例,实际情况下可能需要更多的代码来处理错误处理、用户输入等问题。
from transformers import BertTokenizer, BertForQuestionAnswering import torch # 加载BERT模型和分词器 model_name = 'bert-base-uncased' tokenizer = BertTokenizer.from_pretrained(model_name) model = BertForQuestionAnswering.from_pretrained(model_name) # 输入文本和问题 context = "The Apollo program, also known as Project Apollo, was the third United States human spaceflight program carried out by the National Aeronautics and Space Administration (NASA), which succeeded in landing the first humans on the Moon from 1969 to 1972. Apollo was first conceived during the Eisenhower administration in early 1960 as a follow-up to Project Mercury. It was dedicated to President John F. Kennedy's national goal of landing Americans on the Moon before the end of the 1960s." question = "What was the goal of the Apollo program?" # 对输入进行编码 encoding = tokenizer.encode_plus(question, context, max_length=512, padding='max_length', truncation=True, return_tensors='pt') # 获取输入ids和注意力掩码 input_ids = encoding['input_ids'] attention_mask = encoding['attention_mask'] # 使用BERT模型进行问答 outputs = model(input_ids=input_ids, attention_mask=attention_mask) start_scores = outputs.start_logits end_scores = outputs.end_logits # 获取答案的起始和结束位置 start_index = torch.argmax(start_scores) end_index = torch.argmax(end_scores) # 解码答案 answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(input_ids[0][start_index:end_index+1])) print(answer)
运行这段代码时,你加载了预训练的 BERT 模型和分词器,并对文本和问题进行了编码。然后,你使用 BERT 模型进行问答,通过输入输入ids和注意力掩码,模型输出了起始和结束位置的得分。接着,通过找到得分最高的位置,你解码出了答案,并将其打印出来。
请注意,这段代码还需要安装 Transformers 库和 PyTorch 库,并确保已经下载了预训练的 BERT 模型。
阅读全文
相关推荐
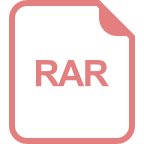
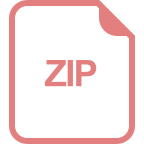
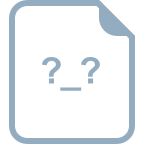
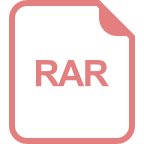
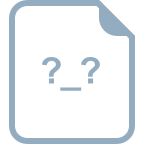
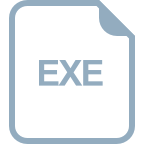
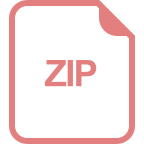
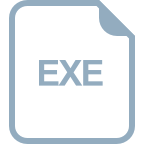
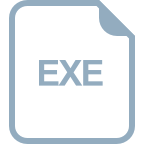
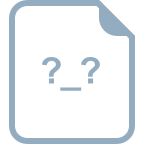

