读取一个存储若干数字的文件,对其中的数字排序后输出的python代码
时间: 2023-06-16 18:05:05 浏览: 165
可以使用Python内置的`sorted`函数对读取文件中的数字进行排序。代码如下:
```python
with open('filename.txt', 'r') as f:
nums = [int(num) for num in f.read().split()] # 读取文件并转换为整数列表
sorted_nums = sorted(nums) # 对数字进行排序
for num in sorted_nums:
print(num) # 输出排序后的数字
```
其中`filename.txt`是存储数字的文件名。`with open('filename.txt', 'r') as f`可以打开文件并自动关闭。`nums`是从文件中读取的数字列表,使用列表推导式实现。`sorted_nums`是排序后的数字列表。最后使用`for`循环遍历输出排序后的数字。
相关问题
编写程序,读取一个存储若干数字的文件,对其中的数字排序后输出python
可以使用Python中的open()函数来读取文件,使用sorted()函数对其中的数字进行排序,再输出即可。具体代码如下:
with open('filename.txt', 'r') as f:
nums = f.read().split() # 读取数字并按空格分割成列表
nums = list(map(int, nums)) # 将数字从字符串转为整数类型
sorted_nums = sorted(nums) # 对数字列表进行排序
for num in sorted_nums:
print(num) # 逐个输出排序后的数字
Python读取一个存储若干数字的文件,对其中的数字排序后输出
可以使用Python的文件操作和排序函数来实现。以下是一个示例代码:
```python
# 打开文件并读取数字
with open('numbers.txt', 'r') as f:
numbers = f.readlines()
# 将数字转换为整数并排序
numbers = [int(num.strip()) for num in numbers]
numbers.sort()
# 输出排序后的数字
for num in numbers:
print(num)
```
假设文件名为 `numbers.txt`,文件内容如下:
```
3
7
1
9
5
```
运行上述代码后,输出结果为:
```
1
3
5
7
9
```
阅读全文
相关推荐
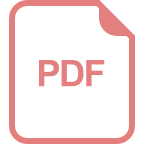













