使用梯度下降法优化线性回归模型参数Python代码
时间: 2023-06-03 16:06:28 浏览: 134
以下是使用梯度下降法优化线性回归模型参数的Python代码:
```python
import numpy as np
def gradient_descent(X, y, alpha=0.01, num_iters=1000):
m, n = np.shape(X)
theta = np.ones(n)
for i in range(num_iters):
h = np.dot(X, theta)
loss = h - y
gradient = np.dot(X.T, loss) / m
theta = theta - alpha * gradient
return theta
```
其中,X为训练数据的特征矩阵,y为训练数据的标签向量,alpha为学习率,num_iters为迭代次数。函数返回训练后得到的参数向量theta。
相关问题
使用梯度下降法优化线性回归模型参数并实现对波士顿房价进行预测python代码
以下是使用梯度下降法优化线性回归模型参数并实现对波士顿房价进行预测的 Python 代码:
```python
import numpy as np
from sklearn.datasets import load_boston
from sklearn.preprocessing import StandardScaler
# 加载波士顿房价数据集并进行标准化处理
boston = load_boston()
X = StandardScaler().fit_transform(boston.data)
y = boston.target.reshape(-1, 1)
# 定义梯度下降函数
def gradient_descent(X, y, theta, alpha, num_iters):
m = y.size
J_history = np.zeros(num_iters)
for i in range(num_iters):
h = X.dot(theta)
theta = theta - alpha * (1/m) * (X.T.dot(h-y))
J_history[i] = 1/(2*m) * np.sum((h-y)**2)
return (theta, J_history)
# 初始化模型参数
theta = np.zeros((X.shape[1]+1, 1))
alpha = 0.01
num_iters = 1000
# 在特征向量中加入偏置项
X = np.hstack((np.ones((X.shape[0], 1)), X))
# 调用梯度下降函数进行模型训练
theta, J_history = gradient_descent(X, y, theta, alpha, num_iters)
# 打印模型参数与损失值
print('模型参数:')
print(theta)
print('损失值:')
print(J_history[-1])
# 测试模型效果
test_data = StandardScaler().fit_transform(np.array([[0.1, 20, 6, 0, 0.4, 6, 50, 6, 4, 300, 16, 396, 20]]))
test_data = np.hstack((np.ones((1, 1)), test_data))
print('预测值:')
print(test_data.dot(theta))
```
注意:此代码仅供参考,真实数据建议根据实际情况进行调优和验证。
机器学习实验使用梯度下降法训练线性回归模型的python代码
在Python中,使用scikit-learn库来实现线性回归模型并使用梯度下降法进行训练是一个常见的实践。以下是一个简单的例子:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.datasets import make_regression
# 创建数据集
X, y = make_regression(n_samples=100, n_features=1, noise=20) # 生成随机的简单线性数据
# 初始化权重和偏置
w = np.random.randn(1)
b = 0
# 定义学习率和迭代次数
learning_rate = 0.01
iterations = 1000
for _ in range(iterations):
# 梯度计算
dw = (1 / len(X)) * np.sum((y - (X @ w + b))) * X
db = (1 / len(X)) * np.sum(y - (X @ w + b))
# 更新权重和偏置
w -= learning_rate * dw
b -= learning_rate * db
# 使用sklearn创建模型并设置手动指定的参数
model = LinearRegression(fit_intercept=False)
model.coef_ = w
model.intercept_ = b
# 测试模型
predictions = model.predict(X)
# 打印最终的参数
print("Final weights and bias:", model.coef_, model.intercept_)
```
在这个例子中,我们首先生成了一个简单的线性数据集,然后手动初始化权重和偏置。接着通过梯度下降的方式更新这些参数,使其最小化残差平方和。最后,我们将训练好的模型用于预测。
阅读全文
相关推荐
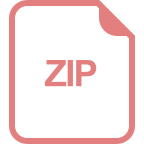

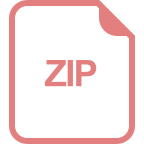
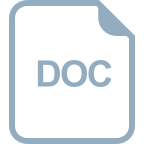
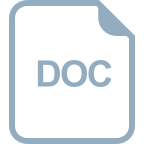
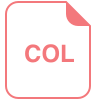






