tensorflow实现resnet模型
时间: 2023-06-29 09:10:53 浏览: 188
ResNet(Residual Network)是由微软亚洲研究院提出的深度残差网络,在ImageNet图像识别比赛中成为了冠军。ResNet通过引入跨层连接(shortcut connection)来缓解了神经网络退化问题,即随着网络层数的增加,模型的性能反而下降。本文将介绍如何使用TensorFlow实现ResNet模型。
首先,我们需要导入必要的库:
```
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, BatchNormalization, ReLU, Add, GlobalAveragePooling2D, Dense
from tensorflow.keras.models import Model
```
然后,我们定义ResNet的基本块(building block):
```
def building_block(x, filters, downsample=False):
residual = x
x = Conv2D(filters=filters, kernel_size=3, strides=1, padding="same")(x)
x = BatchNormalization()(x)
x = ReLU()(x)
x = Conv2D(filters=filters, kernel_size=3, strides=1, padding="same")(x)
x = BatchNormalization()(x)
if downsample:
residual = Conv2D(filters=filters, kernel_size=1, strides=2, padding="same")(residual)
residual = BatchNormalization()(residual)
x = Add()([x, residual])
x = ReLU()(x)
return x
```
上述代码中,`building_block`函数实现了ResNet的基本块。`x`是输入张量,`filters`是卷积核的数量。如果`downsample`为True,则在第一个卷积层后使用步长为2的卷积进行下采样。最后,将输入张量和残差相加,并通过ReLU激活函数进行激活。
接下来,我们定义ResNet的层:
```
def resnet_layer(x, filters, blocks, downsample=False):
x = building_block(x, filters, downsample)
for _ in range(1, blocks):
x = building_block(x, filters)
return x
```
上述代码中,`resnet_layer`函数实现了ResNet的层。`x`是输入张量,`filters`是卷积核的数量,`blocks`是基本块的数量。如果`downsample`为True,则在第一层使用步长为2的卷积进行下采样。接下来,循环调用`building_block`函数来构建基本块。
然后,我们定义ResNet的构建函数:
```
def resnet(input_shape, num_classes):
inputs = Input(shape=input_shape)
x = Conv2D(filters=64, kernel_size=7, strides=2, padding="same")(inputs)
x = BatchNormalization()(x)
x = ReLU()(x)
x = MaxPooling2D(pool_size=3, strides=2, padding="same")(x)
x = resnet_layer(x, filters=64, blocks=2)
x = resnet_layer(x, filters=128, blocks=2, downsample=True)
x = resnet_layer(x, filters=256, blocks=2, downsample=True)
x = resnet_layer(x, filters=512, blocks=2, downsample=True)
x = GlobalAveragePooling2D()(x)
outputs = Dense(units=num_classes, activation="softmax")(x)
model = Model(inputs=inputs, outputs=outputs)
return model
```
上述代码中,`resnet`函数实现了ResNet的构建。`input_shape`是输入张量的形状,`num_classes`是输出类别的数量。首先使用步长为2的7x7卷积进行下采样,然后对特征图进行4个层的残差块构建,并在每个残差块的第一层使用步长为2的卷积进行下采样。最后,通过全局平均池化层和全连接层得到输出结果。
接下来,我们可以使用下面的代码来编译和训练模型:
```
model = resnet(input_shape=(224, 224, 3), num_classes=1000)
model.compile(optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"])
model.fit(x_train, y_train, batch_size=32, epochs=10, validation_data=(x_val, y_val))
```
上述代码中,我们使用ImageNet数据集的训练集和验证集来训练ResNet模型。在训练之前,需要将数据进行预处理,例如将像素值缩放到0到1之间。
阅读全文
相关推荐
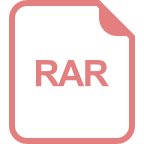
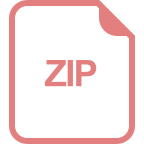
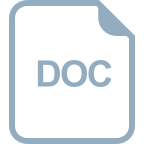
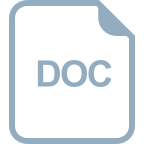


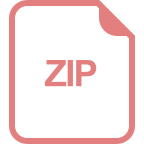
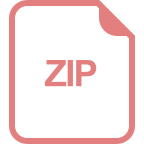
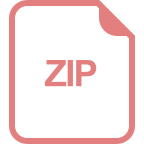

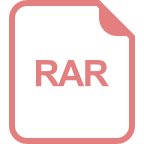
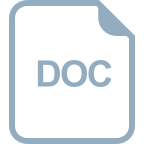
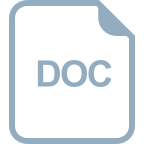




