Vue3中js实现在有限高度中显示列表:列表自动往上滚动显示,鼠标放上去后停止滚动,列表可鼠标滚轮显示剩下的内容,滚动时需要连续滚动,不要出现空白,要求使用vue33组合式api写法实现
时间: 2024-02-17 09:04:35 浏览: 18
好的,以下是使用Vue 3中的组合式API实现在有限高度中显示列表,并实现自动向上滚动、鼠标放上去停止滚动、鼠标滚轮显示剩余内容的示例代码:
```javascript
import { ref, watchEffect } from 'vue';
export default function useScrollList(data, height) {
const containerRef = ref(null);
const showData = ref([]);
let animationId = null;
let scrollTop = 0;
let isHover = false;
const listHeight = () => {
return containerRef.value ? containerRef.value.offsetHeight : 0;
};
const showCount = () => {
return Math.ceil(height / itemHeight);
};
const itemHeight = () => {
return showData.value.length > 0 ? listHeight() / showData.value.length : 0;
};
const startAnimation = () => {
const animate = () => {
scrollTop++;
if (scrollTop + height > showData.value.length * itemHeight()) {
scrollTop = 0;
}
containerRef.value.scrollTop = scrollTop;
animationId = requestAnimationFrame(animate);
};
animationId = requestAnimationFrame(animate);
};
const stopAnimation = () => {
cancelAnimationFrame(animationId);
};
const handleMouseEnter = () => {
isHover = true;
stopAnimation();
};
const handleMouseLeave = () => {
isHover = false;
startAnimation();
};
const handleWheel = (event) => {
event.preventDefault();
const delta = Math.max(-1, Math.min(1, event.deltaY));
scrollTop -= delta * itemHeight();
if (scrollTop < 0) {
scrollTop = 0;
} else if (scrollTop + height > showData.value.length * itemHeight()) {
scrollTop = showData.value.length * itemHeight() - height;
}
containerRef.value.scrollTop = scrollTop;
};
watchEffect(() => {
showData.value = data.slice(-showCount());
if (!isHover) {
startAnimation();
}
});
return {
containerRef,
showData,
handleMouseEnter,
handleMouseLeave,
handleWheel,
};
}
```
在这个示例代码中,我们使用Vue 3中的`ref`和`watchEffect`函数创建了一个响应式的列表容器引用`containerRef`和要显示的数据`showData`。我们还定义了一些变量,如动画ID`animationId`、滚动距离`scrollTop`和鼠标是否悬停在容器上`isHover`等。
接下来,我们定义了一些计算函数,如列表容器的高度`listHeight`、要显示的数据数量`showCount`、每个数据项的高度`itemHeight`等。我们还定义了两个动画函数`startAnimation`和`stopAnimation`,分别用于开始和停止自动滚动的动画。
接着,我们定义了三个事件处理函数`handleMouseEnter`、`handleMouseLeave`和`handleWheel`,分别用于处理鼠标悬停、鼠标移开和滚轮滚动事件。在`handleMouseEnter`和`handleMouseLeave`函数中,我们设置`isHover`变量,并在需要时启动或停止自动滚动的动画。在`handleWheel`函数中,我们计算出滚动的距离,并设置容器的滚动距离。
最后,我们使用`watchEffect`函数监听数据的变化,并根据需要显示对应数量的数据和启动自动滚动的动画。
在组件中,我们可以这样使用上面的示例代码:
```vue
<template>
<div ref="containerRef" @mouseenter="handleMouseEnter" @mouseleave="handleMouseLeave" @wheel="handleWheel" style="height: 200px; overflow: hidden;">
<div v-for="item in showData" :key="item.id">{{ item.text }}</div>
</div>
</template>
<script>
import useScrollList from './useScrollList';
export default {
setup() {
const data = [
{ id: 1, text: '第一条数据' },
{ id: 2, text: '第二条数据' },
{ id: 3, text: '第三条数据' },
// ...
];
const { containerRef, showData, handleMouseEnter, handleMouseLeave, handleWheel } = useScrollList(data, 200);
return {
containerRef,
showData,
handleMouseEnter,
handleMouseLeave,
handleWheel,
};
},
};
</script>
```
在这个示例代码中,我们使用了`useScrollList`函数来处理列表的滚动和显示,并将相关的属性和方法解构出来,以便在模板中使用。我们使用了`ref`函数创建了一个容器引用`containerRef`,并将其绑定到容器上。我们还使用了`v-for`指令循环渲染要显示的数据项。
注意,为了实现滚动时连续滚动,我们需要将容器的`overflow`样式设置为`hidden`。这样就不会出现空白部分。
相关推荐
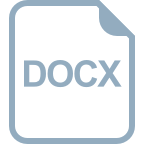
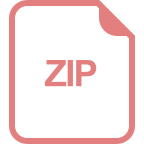
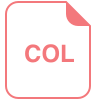
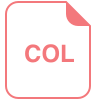
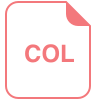
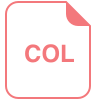
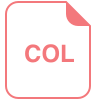










