编一个贪吃蛇代码
时间: 2023-07-24 19:20:30 浏览: 73
好的,以下是一个简单的贪吃蛇代码实现,使用 Python 语言:
```python
import pygame
import random
# 定义常量
WIDTH = 640
HEIGHT = 480
CELL_SIZE = 20
SPEED = 10
# 颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 初始化 Pygame
pygame.init()
# 设置窗口
window = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('贪吃蛇')
# 定义蛇类
class Snake:
def __init__(self):
self.body = [(4, 3), (3, 3), (2, 3)]
self.direction = 'right'
def move(self):
head = self.body[0]
if self.direction == 'up':
new_head = (head[0], head[1] - 1)
elif self.direction == 'down':
new_head = (head[0], head[1] + 1)
elif self.direction == 'left':
new_head = (head[0] - 1, head[1])
elif self.direction == 'right':
new_head = (head[0] + 1, head[1])
self.body.insert(0, new_head)
self.body.pop()
def grow(self):
tail = self.body[-1]
self.body.append(tail)
def is_collide(self):
head = self.body[0]
if head[0] < 0 or head[0] >= WIDTH / CELL_SIZE:
return True
if head[1] < 0 or head[1] >= HEIGHT / CELL_SIZE:
return True
for i in range(1, len(self.body)):
if head == self.body[i]:
return True
return False
def draw(self):
for cell in self.body:
rect = pygame.Rect(cell[0]*CELL_SIZE, cell[1]*CELL_SIZE, CELL_SIZE, CELL_SIZE)
pygame.draw.rect(window, GREEN, rect)
# 定义食物类
class Food:
def __init__(self):
self.position = (random.randint(0, WIDTH/CELL_SIZE-1), random.randint(0, HEIGHT/CELL_SIZE-1))
def draw(self):
rect = pygame.Rect(self.position[0]*CELL_SIZE, self.position[1]*CELL_SIZE, CELL_SIZE, CELL_SIZE)
pygame.draw.rect(window, RED, rect)
# 创建蛇和食物
snake = Snake()
food = Food()
# 游戏循环
clock = pygame.time.Clock()
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and snake.direction != 'down':
snake.direction = 'up'
elif event.key == pygame.K_DOWN and snake.direction != 'up':
snake.direction = 'down'
elif event.key == pygame.K_LEFT and snake.direction != 'right':
snake.direction = 'left'
elif event.key == pygame.K_RIGHT and snake.direction != 'left':
snake.direction = 'right'
# 移动蛇和检测碰撞
snake.move()
if snake.is_collide():
running = False
# 检测是否吃到食物
if snake.body[0] == food.position:
snake.grow()
food = Food()
# 绘制场景
window.fill(BLACK)
snake.draw()
food.draw()
pygame.display.update()
# 控制帧率
clock.tick(SPEED)
# 退出 Pygame
pygame.quit()
exit()
```
注意,这只是一个简单的实现,还有很多细节可以完善,比如增加蛇的长度、速度随着时间增加、死亡动画等。
相关推荐










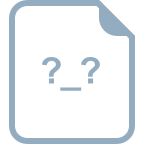
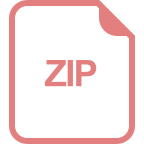
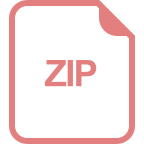