python异步调用发送消息指令
时间: 2023-07-02 16:17:03 浏览: 188
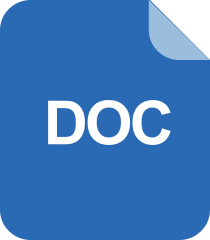
AT指令实现短消息发送

在 Python 中,异步发送消息指令可以使用 asyncio 库来完成。以下是一个简单的示例代码:
```python
import asyncio
async def send_message(message):
# 这里可以编写发送消息的代码,比如使用 requests 库发送 HTTP 请求
# 假设发送消息需要 1 秒钟的时间
await asyncio.sleep(1)
print(f"Message sent: {message}")
async def main():
task1 = asyncio.create_task(send_message("Hello"))
task2 = asyncio.create_task(send_message("World"))
# 等待所有任务完成
await asyncio.gather(task1, task2)
asyncio.run(main())
```
在这个示例中,我们定义了一个 `send_message` 函数来模拟发送消息的过程。由于发送消息可能需要一些时间,我们使用了 `asyncio.sleep` 函数来让程序等待 1 秒钟。然后,在 `main` 函数中,我们使用 `asyncio.create_task` 函数创建了两个任务,并使用 `asyncio.gather` 函数等待所有任务完成。最后,我们使用 `asyncio.run` 函数来运行整个异步程序。
阅读全文
相关推荐
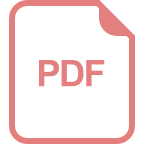
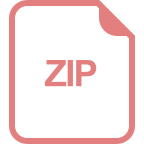









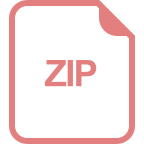
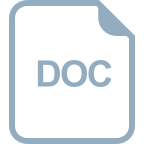
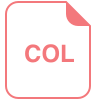
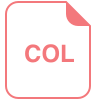
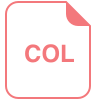
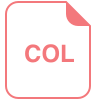