使用des算法实现对学号姓名加密java代码
时间: 2023-07-30 07:07:18 浏览: 181
好的,以下是使用DES算法对学号姓名进行加密的Java代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.DESKeySpec;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.SecretKeyFactory;
import javax.crypto.SecretKey;
public class DESUtil {
private static final String KEY = "12345678"; // 密钥
private static final String IV = "12345678"; // 初始向量
public static String encrypt(String source) throws Exception {
DESKeySpec keySpec = new DESKeySpec(KEY.getBytes("UTF-8"));
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey key = keyFactory.generateSecret(keySpec);
IvParameterSpec iv = new IvParameterSpec(IV.getBytes("UTF-8"));
Cipher cipher = Cipher.getInstance("DES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, key, iv);
byte[] encrypted = cipher.doFinal(source.getBytes("UTF-8"));
return bytesToHexString(encrypted);
}
public static String decrypt(String encrypted) throws Exception {
byte[] enc = hexStringToBytes(encrypted);
DESKeySpec keySpec = new DESKeySpec(KEY.getBytes("UTF-8"));
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey key = keyFactory.generateSecret(keySpec);
IvParameterSpec iv = new IvParameterSpec(IV.getBytes("UTF-8"));
Cipher cipher = Cipher.getInstance("DES/CBC/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, key, iv);
byte[] decrypted = cipher.doFinal(enc);
return new String(decrypted, "UTF-8");
}
private static String bytesToHexString(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < bytes.length; i++) {
String hex = Integer.toHexString(bytes[i] & 0xFF);
if (hex.length() < 2) {
sb.append('0');
}
sb.append(hex);
}
return sb.toString();
}
private static byte[] hexStringToBytes(String hexString) {
if (hexString == null || hexString.equals("")) {
return null;
}
hexString = hexString.toUpperCase();
int length = hexString.length() / 2;
char[] hexChars = hexString.toCharArray();
byte[] d = new byte[length];
for (int i = 0; i < length; i++) {
int pos = i * 2;
d[i] = (byte) (charToByte(hexChars[pos]) << 4 | charToByte(hexChars[pos + 1]));
}
return d;
}
private static byte charToByte(char c) {
return (byte) "0123456789ABCDEF".indexOf(c);
}
}
```
以上代码使用了Java标准库中的DES算法实现加密和解密。加密和解密过程中使用了PKCS5填充,以保证数据块长度为8的倍数。需要注意的是,由于DES算法已经被认为是不安全的算法,因此在实际应用中需要使用更加安全的算法来进行数据加密和解密。
阅读全文
相关推荐
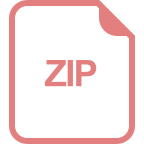






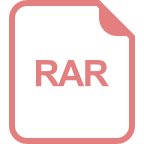








