QT 使用hiRedis获取第一层以:分隔符所有key,并返回key名称和key下面有多少个key
时间: 2024-04-05 18:35:29 浏览: 71
好的,可以使用以下代码实现:
```cpp
#include <iostream>
#include <hiredis/hiredis.h>
#include <QStringList>
int main(int argc, char *argv[])
{
// 连接 Redis
redisContext *context = redisConnect("127.0.0.1", 6379);
if (context == nullptr || context->err) {
if (context) {
std::cout << "Error: " << context->errstr << std::endl;
redisFree(context);
} else {
std::cout << "Can't allocate redis context." << std::endl;
}
return EXIT_FAILURE;
}
// 发送 Redis 命令
redisReply *reply = static_cast<redisReply*>(redisCommand(context, "SCAN 0 MATCH * COUNT 1000"));
if (reply == nullptr) {
std::cout << "Error: Failed to execute Redis command." << std::endl;
redisFree(context);
return EXIT_FAILURE;
}
// 解析 Redis 命令的返回值
if (reply->type == REDIS_REPLY_ARRAY) {
if (reply->elements == 2 && reply->element[0]->type == REDIS_REPLY_STRING && reply->element[1]->type == REDIS_REPLY_ARRAY) {
std::cout << "The key names and the number of keys under them:\n";
for (size_t i = 0; i < reply->element[1]->elements; i++) {
const char *key = reply->element[1]->element[i]->str;
std::cout << key << ": ";
// 获取子 key 的数量
redisReply *subReply = static_cast<redisReply*>(redisCommand(context, "DBSIZE %s*", key));
if (subReply == nullptr) {
std::cout << "Error: Failed to execute Redis command." << std::endl;
redisFree(context);
return EXIT_FAILURE;
}
if (subReply->type == REDIS_REPLY_INTEGER) {
std::cout << subReply->integer << std::endl;
} else {
std::cout << "Error: Invalid Redis reply type." << std::endl;
}
freeReplyObject(subReply);
}
} else {
std::cout << "Error: Invalid Redis reply array elements." << std::endl;
}
} else {
std::cout << "Error: Invalid Redis reply type." << std::endl;
}
// 释放资源
freeReplyObject(reply);
redisFree(context);
return EXIT_SUCCESS;
}
```
这段代码使用 SCAN 命令遍历 Redis 中的所有 key,然后使用 DBSIZE 命令获取每个 key 下的子 key 数量。在输出结果时,将 key 名称和子 key 数量一并输出即可。
阅读全文
相关推荐
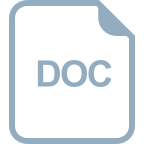
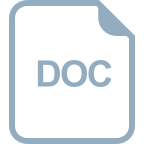
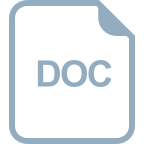



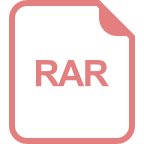
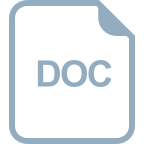
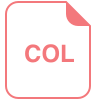
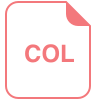
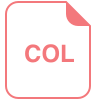
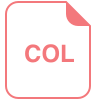
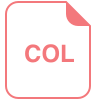
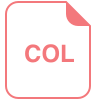
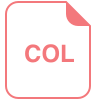



