import requests from lxml import etree url="https://www.80142.com/txt/82776.html" html=requests.get(url) print(html)出现requests not get
时间: 2023-06-26 09:09:04 浏览: 67
这个问题可能是因为您没有正确安装 requests 库。请尝试在命令行中使用以下命令安装 requests 库:
```
pip install requests
```
如果您已经安装了 requests 库,那么可能是因为您的代码中出现了其他问题。请检查您的代码是否正确导入了 requests 库,并且 URL 是否正确。另外,您的代码中缺少换行符,请注意格式。下面是您的代码的修改版:
```
import requests
from lxml import etree
url = "https://www.80142.com/txt/82776.html"
html = requests.get(url)
print(html)
```
相关问题
解决一下以下代码报错的import requests from lxml import etree url = 'https://www.shanghairanking.cn/institution' headers = { 'User-Agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36' } res = requests.get(url=url,headers=headers) print(res.status_code) html = etree.HTML(res.text) lis = html.xpath("//*[@id="__layout"]/div/div[2]/div[2]/div[1]") print(len(lis))
这段代码有一个小错误,就是在xpath的语句中双引号没有转义,需要改成单引号。修改后的代码如下:
```python
import requests
from lxml import etree
url = 'https://www.shanghairanking.cn/institution'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36'
}
res = requests.get(url=url, headers=headers)
print(res.status_code)
html = etree.HTML(res.text)
lis = html.xpath('//*[@id="__layout"]/div/div[2]/div[2]/div[1]')
print(len(lis))
```
这样就可以正确运行了,输出页面中指定元素的数量。
import requests from lxml import etree url = "https://www.chzc.edu.cn/info/1355/80006.htm" web = requests.get(url) web.encoding = "utf-8" xp = etree.HTML(web.text) imgurls = xp.xpath('//*[@id="vsb_content_1011"]/p/img/@src') print(imgurls) realurls = [] for url in imgurls: realurls.append("https://www.chzc.edu.cn/"+url) print(realurls) for url in realurls: imgdata = requests.get(url) with open("c:\\"++".jpg","wb")as file: file.write(imgdata.content)报错原因
报错原因是因为代码中第10行的文件名拼接出现问题,文件名应该是字符串类型,而代码中缺少字符串连接符号。可以将该行代码修改为:
```
with open("c:\\" + "filename" + ".jpg", "wb") as file:
```
其中的 "filename" 可以替换为你想要的图片文件名。另外,还需要注意转义字符 "\" 需要使用双反斜杠 "\\" 表示。
相关推荐
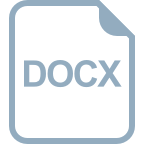
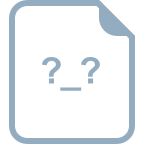
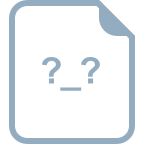












