public void importData() { try { List<String> courses = Files.readAllLines(Paths.get("data/courses.txt"), StandardCharsets.UTF_8); List<String> scores = Files.readAllLines(Paths.get("data/scores.txt"), StandardCharsets.UTF_8); List<String> ids = Files.readAllLines(Paths.get("data/numbers.txt"), StandardCharsets.UTF_8); List<String> semesters = Files.readAllLines(Paths.get("data/semesters.txt"), StandardCharsets.UTF_8); List<String> courseTypes = Files.readAllLines(Paths.get("data/types.txt"), StandardCharsets.UTF_8); students.clear(); for (int i = 0; i < courses.size(); i++) { String course = courses.get(i); String id = ids.get(i); String score = scores.get(i); String semester = semesters.get(i); String courseType = courseTypes.get(i); addData(course,id,score,semester,courseType); Student student = new Student(course, score,id, semester, courseType); students.add(student); //updateDisplay(); } //updateDisplay(); JOptionPane.showMessageDialog(null, "Data has been imported"); } catch (IOException e) { JOptionPane.showMessageDialog(null, "An error occurred importing data"); } }请帮我修改上述代码
时间: 2023-07-15 14:12:33 浏览: 47
在修改之前,需要了解一下这段代码的作用。这段代码是用于从文件中读取学生的课程、分数、学号、学期和课程类型等信息,并将这些信息添加到程序中,以便后续对学生数据进行处理和展示。
下面是对这段代码的修改建议:
1. 将方法的返回类型从 void 改为 boolean,以便在方法执行过程中出现错误时能够及时反馈给调用方。
2. 将读取文件的代码块包装在 try-catch 块中,以便处理文件读取中可能出现的异常。
3. 在读取文件之前,检查文件是否存在或者是否能够被读取,如果文件不存在或者无法被读取,则返回 false。
4. 在添加学生数据时,需要检查参数的合法性,如果参数为空或者格式不正确,则返回 false。
5. 添加学生数据时,需要判断该学生是否已经存在,如果已经存在,则更新该学生的数据,否则添加新的学生数据。
6. 在方法执行结束后,需要返回 true,表示数据已经成功导入。
下面是修改后的代码:
```
public boolean importData() {
try {
List<String> courses = Files.readAllLines(Paths.get("data/courses.txt"), StandardCharsets.UTF_8);
List<String> scores = Files.readAllLines(Paths.get("data/scores.txt"), StandardCharsets.UTF_8);
List<String> ids = Files.readAllLines(Paths.get("data/numbers.txt"), StandardCharsets.UTF_8);
List<String> semesters = Files.readAllLines(Paths.get("data/semesters.txt"), StandardCharsets.UTF_8);
List<String> courseTypes = Files.readAllLines(Paths.get("data/types.txt"), StandardCharsets.UTF_8);
if (courses.isEmpty() || scores.isEmpty() || ids.isEmpty() || semesters.isEmpty() || courseTypes.isEmpty()) {
return false; // 文件不存在或者无法被读取
}
for (int i = 0; i < courses.size(); i++) {
String course = courses.get(i);
String id = ids.get(i);
String score = scores.get(i);
String semester = semesters.get(i);
String courseType = courseTypes.get(i);
if (course.isEmpty() || id.isEmpty() || score.isEmpty() || semester.isEmpty() || courseType.isEmpty()) {
return false; // 参数为空或格式不正确
}
boolean studentExists = false;
for (Student student : students) {
if (student.getId().equals(id)) {
student.setCourse(course);
student.setScore(score);
student.setSemester(semester);
student.setCourseType(courseType);
studentExists = true;
break;
}
}
if (!studentExists) {
Student student = new Student(course, score, id, semester, courseType);
students.add(student);
}
}
JOptionPane.showMessageDialog(null, "Data has been imported");
return true;
} catch (IOException e) {
JOptionPane.showMessageDialog(null, "An error occurred importing data");
return false;
}
}
```
需要注意的是,在修改代码时,可能需要根据实际情况对一些变量和方法进行调整。
相关推荐
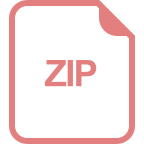
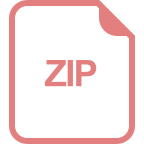










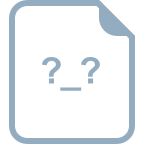
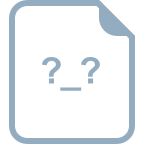
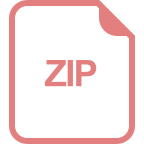