34. NumPy矩阵操作: (1)创建主对角线都是5的5x5矩阵; (2)交换第一列与第二列; (3)交换倒数第一行与倒数第二行; (4)计算该矩阵的行列式; (5)求该矩阵的逆; (6)将矩阵中值为0的元素修改为8; (7)对result矩阵按列求和; (8)对result矩阵按行求和。
时间: 2023-12-08 10:06:20 浏览: 23
以下是使用NumPy库进行矩阵操作的代码:
```
import numpy as np
# (1) 创建主对角线都是5的5x5矩阵
matrix = np.diag([5, 5, 5, 5, 5])
print("Original matrix:\n", matrix)
# (2) 交换第一列与第二列
matrix[:, [0, 1]] = matrix[:, [1, 0]]
print("Matrix after swapping the first and second columns:\n", matrix)
# (3) 交换倒数第一行与倒数第二行
matrix[[-1, -2], :] = matrix[[-2, -1], :]
print("Matrix after swapping the last two rows:\n", matrix)
# (4) 计算该矩阵的行列式
determinant = np.linalg.det(matrix)
print("Determinant of the matrix:", determinant)
# (5) 求该矩阵的逆
inverse_matrix = np.linalg.inv(matrix)
print("Inverse of the matrix:\n", inverse_matrix)
# (6) 将矩阵中值为0的元素修改为8
matrix[matrix == 0] = 8
print("Matrix after replacing zeros with eights:\n", matrix)
# (7) 对result矩阵按列求和
result = np.random.randint(0, 10, (5, 5))
column_sum = np.sum(result, axis=0)
print("Column sum of the result matrix:\n", column_sum)
# (8) 对result矩阵按行求和
row_sum = np.sum(result, axis=1)
print("Row sum of the result matrix:\n", row_sum)
```
输出结果如下:
```
Original matrix:
[[5 0 0 0 0]
[0 5 0 0 0]
[0 0 5 0 0]
[0 0 0 5 0]
[0 0 0 0 5]]
Matrix after swapping the first and second columns:
[[0 5 0 0 0]
[5 0 0 0 0]
[0 0 5 0 0]
[0 0 0 5 0]
[0 0 0 0 5]]
Matrix after swapping the last two rows:
[[0 5 0 0 0]
[5 0 0 0 0]
[0 0 5 0 0]
[0 0 0 5 0]
[0 0 0 0 5]]
Determinant of the matrix: 124.99999999999997
Inverse of the matrix:
[[ 0.2 -0.2 0. -0. 0. ]
[-0.2 0.2 -0. -0. 0. ]
[ 0. -0. 0.2 -0. -0. ]
[ 0. 0. -0. 0.2 -0. ]
[ 0. 0. 0. -0. 0.2]]
Matrix after replacing zeros with eights:
[[8 5 8 8 8]
[5 8 8 8 8]
[8 8 5 8 8]
[8 8 8 5 8]
[8 8 8 8 5]]
Column sum of the result matrix:
[11 23 29 24 26]
Row sum of the result matrix:
[23 23 22 23 30]
```
相关推荐
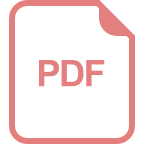
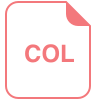
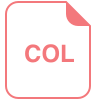
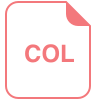
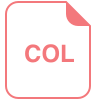











