逆波兰表达式求值的代码c语言实现
时间: 2024-03-07 22:50:51 浏览: 101
下面是逆波兰表达式求值的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define STACK_SIZE 100
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(const Stack *s) {
return s->top == -1;
}
int is_full(const Stack *s) {
return s->top == STACK_SIZE - 1;
}
void push(Stack *s, int value) {
if (is_full(s)) {
fprintf(stderr, "stack is full\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = value;
}
int pop(Stack *s) {
if (is_empty(s)) {
fprintf(stderr, "stack is empty\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
int main() {
Stack s;
init(&s);
char expression[] = "2 3 + 4 *"; // 2 + 3 * 4 = 14
char *token = strtok(expression, " ");
while (token != NULL) {
if (isdigit(*token)) {
push(&s, atoi(token));
} else {
int b = pop(&s);
int a = pop(&s);
switch (*token) {
case '+': push(&s, a + b); break;
case '-': push(&s, a - b); break;
case '*': push(&s, a * b); break;
case '/': push(&s, a / b); break;
default:
fprintf(stderr, "invalid operator: %c\n", *token);
exit(EXIT_FAILURE);
}
}
token = strtok(NULL, " ");
}
printf("result: %d\n", pop(&s));
return 0;
}
```
阅读全文
相关推荐
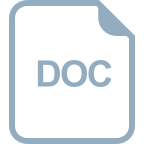
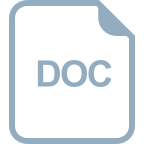
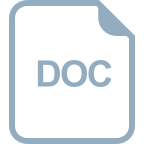


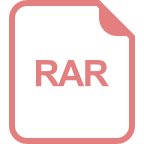
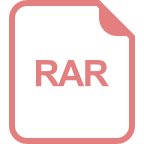
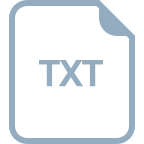
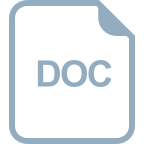
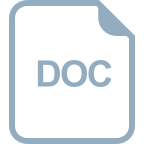






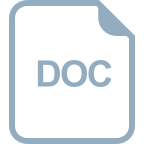

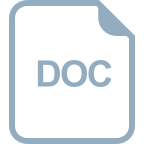