python简单程序运用turtle画图以如图格式绘制7段数码管,输出当前时间
时间: 2023-08-05 12:22:17 浏览: 102
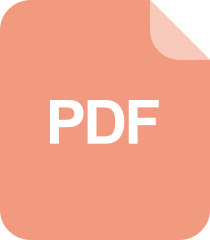
python使用turtle库绘制时钟

好的,以下是Python程序以7段数码管格式绘制当前时间的代码,使用turtle库绘制:
```python
import turtle
import time
# 7段数码管的编码
digits = {
0: ['1111110', '1010000', '1010000', '1010000', '1010000', '1010000', '1111110'],
1: ['0100100', '0010100', '0010100', '0010100', '0010100', '0010100', '0111110'],
2: ['1111110', '0000010', '0000010', '1111110', '1000000', '1000000', '1111110'],
3: ['1111110', '0000010', '0000010', '1111110', '0000010', '0000010', '1111110'],
4: ['1010000', '1010000', '1010000', '1111110', '0000010', '0000010', '0000010'],
5: ['1111110', '1000000', '1000000', '1111110', '0000010', '0000010', '1111110'],
6: ['1111110', '1000000', '1000000', '1111110', '1010000', '1010000', '1111110'],
7: ['1111110', '0000010', '0000010', '0000010', '0000010', '0000010', '0000010'],
8: ['1111110', '1010000', '1010000', '1111110', '1010000', '1010000', '1111110'],
9: ['1111110', '1010000', '1010000', '1111110', '0000010', '0000010', '1111110']
}
def draw_line(x, y, length, angle):
turtle.penup()
turtle.goto(x, y)
turtle.setheading(angle)
turtle.pendown()
turtle.forward(length)
def draw_segment(x, y, width, height, segments):
draw_line(x, y, width, 0)
draw_line(x, y, height, 90)
draw_line(x + width, y, height, 90)
draw_line(x, y + width, height, 90)
draw_line(x, y + width, width, 0)
draw_line(x, y + height, width, 0)
draw_line(x + height, y + width, width, 0)
if segments[0] == '1':
draw_line(x, y, width, 0)
if segments[1] == '1':
draw_line(x, y + width, width, 0)
if segments[2] == '1':
draw_line(x, y, height, 90)
if segments[3] == '1':
draw_line(x + height, y, height, 90)
if segments[4] == '1':
draw_line(x, y + height, width, 0)
if segments[5] == '1':
draw_line(x + height, y + width, width, 0)
if segments[6] == '1':
draw_line(x, y + width, height, 90)
def draw_digit(digit, x, y, width, height):
segments = digits[digit]
draw_segment(x, y, width, height, segments[0])
draw_segment(x + width, y + height, height, width, segments[1])
draw_segment(x + width, y + 2 * height + width, height, width, segments[2])
draw_segment(x, y + 2 * height + 2 * width, width, height, segments[3])
draw_segment(x, y + height + width, height, width, segments[4])
draw_segment(x, y + height, height, width, segments[5])
draw_segment(x, y + 2 * height, width, height, segments[6])
while True:
# 获取当前时间
current_time = time.strftime("%H:%M:%S", time.localtime())
# 清屏
turtle.reset()
# 输出当前时间
x = -300
y = 0
width = 50
height = 100
for i in range(len(current_time)):
if current_time[i].isdigit():
draw_digit(int(current_time[i]), x, y, width, height)
else:
if current_time[i] == ':':
draw_segment(x + width, y + height, width, height, '0000100')
draw_segment(x + width, y + 2 * height + width, width, height, '0000100')
else:
pass
x += 2 * (width + height)
# 更新画面
turtle.update()
# 暂停1秒
time.sleep(1)
turtle.done()
```
这个程序会在窗口中以7段数码管的形式输出当前时间,并且每隔1秒钟会自动更新一次。
阅读全文
相关推荐
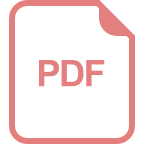









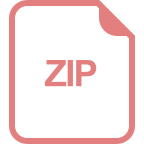