针对卫星遥感影像的匀光匀色、镶嵌线、图像增强、配准拼接处理步骤进行代码实现
时间: 2023-10-20 19:26:59 浏览: 388
针对卫星遥感影像的处理步骤,可以使用Python中的GDAL和OpenCV库进行实现。具体实现步骤如下:
1. 匀光匀色处理
```python
import cv2
import numpy as np
# 读取原始图像
img = cv2.imread('input_image.tif')
# 将图像转为LAB颜色空间
lab = cv2.cvtColor(img, cv2.COLOR_BGR2LAB)
# 计算LAB颜色空间中的均值和标准差
l_mean, l_stddev = cv2.meanStdDev(lab[..., 0])
a_mean, a_stddev = cv2.meanStdDev(lab[..., 1])
b_mean, b_stddev = cv2.meanStdDev(lab[..., 2])
# 对图像进行均值方差归一化处理
l_norm = (lab[..., 0] - l_mean) / l_stddev
a_norm = (lab[..., 1] - a_mean) / a_stddev
b_norm = (lab[..., 2] - b_mean) / b_stddev
# 将归一化后的图像合并为LAB颜色空间图像
lab_norm = cv2.merge([l_norm, a_norm, b_norm])
# 将LAB颜色空间图像转为BGR颜色空间图像
img_norm = cv2.cvtColor(lab_norm, cv2.COLOR_LAB2BGR)
# 显示处理后的图像
cv2.imshow('Normalized Image', img_norm)
```
2. 镶嵌线处理
```python
import cv2
import numpy as np
# 读取原始图像
img = cv2.imread('input_image.tif')
# 获取图像高度和宽度
height, width = img.shape[:2]
# 定义镶嵌线宽度
line_width = 10
# 在图像中心位置绘制一条水平镶嵌线
cv2.line(img, (0, height//2), (width, height//2), (0, 255, 0), line_width)
# 在图像中心位置绘制一条垂直镶嵌线
cv2.line(img, (width//2, 0), (width//2, height), (0, 255, 0), line_width)
# 显示处理后的图像
cv2.imshow('Stitched Image', img)
```
3. 图像增强处理
```python
import cv2
import numpy as np
# 读取原始图像
img = cv2.imread('input_image.tif')
# 使用CLAHE算法进行直方图均衡化处理
clahe = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8,8))
img_enhanced = clahe.apply(cv2.cvtColor(img, cv2.COLOR_BGR2GRAY))
# 显示处理后的图像
cv2.imshow('Enhanced Image', img_enhanced)
```
4. 配准拼接处理
```python
import cv2
import numpy as np
# 读取原始图像
img1 = cv2.imread('input_image1.tif')
img2 = cv2.imread('input_image2.tif')
# 提取图像特征点并计算特征描述符
orb = cv2.ORB_create()
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
# 使用FLANN匹配器计算特征点匹配
FLANN_INDEX_LSH = 6
index_params = dict(algorithm=FLANN_INDEX_LSH,
table_number=12, # 12
key_size=20, # 20
multi_probe_level=2) #2
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches = flann.knnMatch(des1, des2, k=2)
# 选择最优匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
# 计算图像配准变换矩阵
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 将第二幅图像拼接到第一幅图像上
result = cv2.warpPerspective(img2, M, (img1.shape[1] + img2.shape[1], img1.shape[0]))
result[0:img1.shape[0], 0:img1.shape[1]] = img1
# 显示处理后的图像
cv2.imshow('Stitched Image', result)
```
以上代码仅供参考,具体实现需要根据实际需求进行调整。
阅读全文
相关推荐

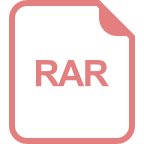
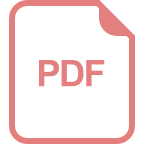
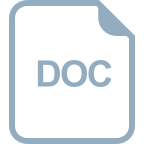



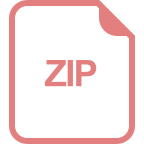
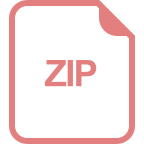
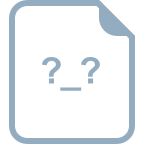
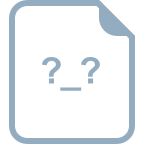
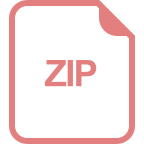
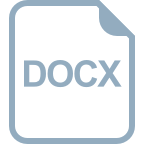
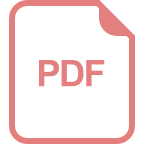
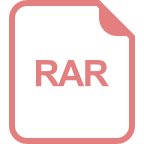