数据结构表达式求值c语言代码,要求以输入=为结束
时间: 2024-05-01 17:16:51 浏览: 20
以下是一个基于栈的表达式求值C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef enum { false, true } bool;
typedef struct {
int top;
int data[MAX_STACK_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
bool is_empty(Stack *s) {
return s->top == -1;
}
bool is_full(Stack *s) {
return s->top == MAX_STACK_SIZE - 1;
}
void push(Stack *s, int item) {
if (is_full(s)) {
printf("Error: stack is full\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = item;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Error: stack is empty\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
int peek(Stack *s) {
if (is_empty(s)) {
printf("Error: stack is empty\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
int evaluate(char *expression) {
Stack operand_stack;
Stack operator_stack;
init(&operand_stack);
init(&operator_stack);
while (*expression != '=') {
if (isdigit(*expression)) {
int operand = 0;
while (isdigit(*expression)) {
operand = operand * 10 + (*expression - '0');
expression++;
}
push(&operand_stack, operand);
} else if (*expression == '(') {
push(&operator_stack, '(');
expression++;
} else if (*expression == ')') {
while (peek(&operator_stack) != '(') {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
switch (op) {
case '+':
push(&operand_stack, op1 + op2);
break;
case '-':
push(&operand_stack, op1 - op2);
break;
case '*':
push(&operand_stack, op1 * op2);
break;
case '/':
push(&operand_stack, op1 / op2);
break;
}
}
pop(&operator_stack);
expression++;
} else if (*expression == '+' || *expression == '-') {
while (!is_empty(&operator_stack) && peek(&operator_stack) != '(') {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
switch (op) {
case '+':
push(&operand_stack, op1 + op2);
break;
case '-':
push(&operand_stack, op1 - op2);
break;
case '*':
push(&operand_stack, op1 * op2);
break;
case '/':
push(&operand_stack, op1 / op2);
break;
}
}
push(&operator_stack, *expression);
expression++;
} else if (*expression == '*' || *expression == '/') {
while (!is_empty(&operator_stack) && peek(&operator_stack) != '(' && peek(&operator_stack) != '+' && peek(&operator_stack) != '-') {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
switch (op) {
case '+':
push(&operand_stack, op1 + op2);
break;
case '-':
push(&operand_stack, op1 - op2);
break;
case '*':
push(&operand_stack, op1 * op2);
break;
case '/':
push(&operand_stack, op1 / op2);
break;
}
}
push(&operator_stack, *expression);
expression++;
} else {
printf("Error: invalid input\n");
exit(EXIT_FAILURE);
}
}
while (!is_empty(&operator_stack)) {
int op2 = pop(&operand_stack);
int op1 = pop(&operand_stack);
char op = pop(&operator_stack);
switch (op) {
case '+':
push(&operand_stack, op1 + op2);
break;
case '-':
push(&operand_stack, op1 - op2);
break;
case '*':
push(&operand_stack, op1 * op2);
break;
case '/':
push(&operand_stack, op1 / op2);
break;
}
}
return pop(&operand_stack);
}
int main() {
char expression[MAX_STACK_SIZE];
printf("Enter an expression (use = to end): ");
fgets(expression, MAX_STACK_SIZE, stdin);
int result = evaluate(expression);
printf("Result: %d\n", result);
return 0;
}
```
该代码使用两个栈:操作数栈和操作符栈。当遇到数字时,将其推入操作数栈;当遇到左括号时,将其推入操作符栈;当遇到右括号时,从操作数栈中弹出两个操作数,从操作符栈中弹出一个操作符,并将计算结果推入操作数栈;当遇到加、减、乘、除号时,比较其优先级,并根据优先级决定是否从栈中弹出操作数和操作符,并将计算结果推入操作数栈。最后,将操作符栈中剩余的操作符逐个弹出,并按照上述规则计算结果。
相关推荐
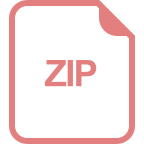
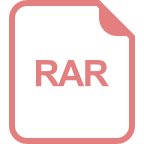
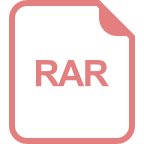














