用pytorch写一个LSTM网络结构,其中不允许使用nn.LSTM方法
时间: 2023-07-20 13:26:45 浏览: 48
以下是使用 PyTorch 实现 LSTM 网络的代码示例,其中不使用 `nn.LSTM` 方法:
```python
import torch
import torch.nn as nn
class MyLSTM(nn.Module):
def __init__(self, input_size, hidden_size, num_layers):
super(MyLSTM, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.num_layers = num_layers
# 定义 LSTM 层的参数
self.weight_ih = nn.Parameter(torch.Tensor(input_size, 4 * hidden_size))
self.weight_hh = nn.Parameter(torch.Tensor(hidden_size, 4 * hidden_size))
self.bias = nn.Parameter(torch.Tensor(4 * hidden_size))
# 初始化 LSTM 层的参数
self.reset_parameters()
def reset_parameters(self):
# 初始化 LSTM 层的参数
std = 1.0 / ((self.hidden_size + self.input_size) ** 0.5)
for weight in self.parameters():
nn.init.uniform_(weight, -std, std)
def forward(self, x):
# 初始化 LSTM 隐藏状态和单元状态
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
# 定义 LSTM 层的前向传播过程
gates = x @ self.weight_ih + h0[0] @ self.weight_hh + self.bias
ingate, forgetgate, cellgate, outgate = gates.chunk(4, 1)
ingate = torch.sigmoid(ingate)
forgetgate = torch.sigmoid(forgetgate)
cellgate = torch.tanh(cellgate)
outgate = torch.sigmoid(outgate)
cy = (forgetgate * c0) + (ingate * cellgate)
hy = outgate * torch.tanh(cy)
# 返回 LSTM 网络的输出
return hy
```
在这个代码示例中,我们定义了一个名为 `MyLSTM` 的继承自 `nn.Module` 的类,它包含了一个 LSTM 层。在类的初始化函数中,我们定义了 LSTM 层的输入维度 `input_size`、隐藏状态的维度 `hidden_size`,以及 LSTM 层的层数 `num_layers`。然后,我们使用 `nn.Parameter()` 函数定义了 LSTM 层的权重张量 `weight_ih` 和 `weight_hh`,以及偏置张量 `bias`,并将它们保存在类中。接着,我们调用了 `reset_parameters()` 函数来初始化这些参数。
在前向传播函数中,我们首先初始化了 LSTM 的隐藏状态和单元状态 `h0` 和 `c0`,并将其转移到输入张量 `x` 所在的设备上。然后,我们使用输入张量 `x`、隐藏状态 `h0[0]` 和 LSTM 层的权重张量 `weight_ih` 和 `weight_hh`,以及偏置张量 `bias` 计算了 LSTM 层的门控值和单元状态。最后,我们使用门控值和单元状态计算了 LSTM 的输出张量 `hy`。注意,在这个实现中,我们只计算了一个时间步的前向传播,因此这个 LSTM 网络只适用于序列长度为 1 的情况。
使用这个代码示例,我们可以创建一个 `MyLSTM` 对象,将输入张量传递给它,然后使用它来进行前向传播。
相关推荐
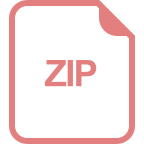
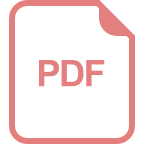
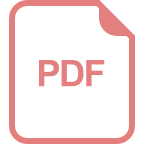














