交通标志的特征提取部分为什么使用ResNet模型?
时间: 2023-06-17 16:05:37 浏览: 83
交通标志的特征提取是一个图像分类问题,而ResNet模型是在图像分类任务中表现非常优秀的深度学习模型之一。其特点是可以训练非常深的神经网络,并且在深层次网络中解决了梯度消失和梯度爆炸的问题,使得网络可以更好地学习到图像的特征。因此,使用ResNet模型作为交通标志特征提取的模型,可以更好地提取图像中的特征,从而提高分类准确率。此外,ResNet模型已经在许多计算机视觉任务中得到了广泛应用,具有很好的可迁移性和通用性,因此也可以很方便地应用于交通标志识别任务。
相关问题
请给出使用Faster R-CNN获取图像中交通标志的位置,并使用ResNet提取交通标志区域特征,再通过一个卷积神经网络实现对交通标志分类的详细代码
好的,以下是使用Faster R-CNN获取图像中交通标志位置的代码:
```python
# 导入必要的库
import torch
import torchvision
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 加载训练好的 Faster R-CNN 模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# 设置为评估模式
model.eval()
# 定义标签和颜色
labels = [
'speed limit 20',
'speed limit 30',
'speed limit 50',
'speed limit 60',
'speed limit 70',
'speed limit 80',
'end of speed limit 80',
'speed limit 100',
'speed limit 120',
'no passing',
'no passing for vehicles over 3.5 metric tons',
'right-of-way at the next intersection',
'priority road',
'yield',
'stop',
'no vehicles',
'vehicles over 3.5 metric tons prohibited',
'no entry',
'general caution',
'dangerous curve to the left',
'dangerous curve to the right',
'double curve',
'bumpy road',
'slippery road',
'road narrows on the right',
'road work',
'traffic signals',
'pedestrians',
'children crossing',
'bicycles crossing',
'beware of ice/snow',
'wild animals crossing',
'end of all speed and passing limits',
'turn right ahead',
'turn left ahead',
'ahead only',
'go straight or right',
'go straight or left',
'keep right',
'keep left',
'roundabout mandatory',
'end of no passing',
'end of no passing by vehicles over 3.5 metric tons'
]
colors = np.random.uniform(0, 255, size=(len(labels), 3))
# 加载测试图像
image = cv2.imread('test.jpg')
# 转换为 PyTorch 张量
image = torch.from_numpy(image / 255.0).float().permute(2, 0, 1)
# 将图像放入模型中进行预测
with torch.no_grad():
predictions = model([image])
# 获取预测结果
boxes = predictions[0]['boxes']
scores = predictions[0]['scores']
labels = predictions[0]['labels']
# 设置阈值,过滤掉低置信度的预测结果
threshold = 0.5
boxes = boxes[scores >= threshold]
labels = labels[scores >= threshold]
# 将预测结果绘制在图像上
image = cv2.imread('test.jpg')
for box, label in zip(boxes, labels):
x1, y1, x2, y2 = box.cpu().numpy().astype(np.int32)
color = colors[label]
cv2.rectangle(image, (x1, y1), (x2, y2), color, 2)
cv2.putText(image, labels[label], (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, color, 2)
# 显示图像
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()
```
接下来是使用 ResNet 提取交通标志区域特征和使用卷积神经网络实现交通标志分类的代码:
```python
# 导入必要的库
import torch
import torchvision
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 加载训练好的 Faster R-CNN 模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# 设置为评估模式
model.eval()
# 加载训练好的交通标志分类模型
classifier = torchvision.models.resnet18(pretrained=True)
classifier.fc = torch.nn.Linear(classifier.fc.in_features, 43)
classifier.load_state_dict(torch.load('classifier.pth'))
classifier.eval()
# 定义标签和颜色
labels = [
'speed limit 20',
'speed limit 30',
'speed limit 50',
'speed limit 60',
'speed limit 70',
'speed limit 80',
'end of speed limit 80',
'speed limit 100',
'speed limit 120',
'no passing',
'no passing for vehicles over 3.5 metric tons',
'right-of-way at the next intersection',
'priority road',
'yield',
'stop',
'no vehicles',
'vehicles over 3.5 metric tons prohibited',
'no entry',
'general caution',
'dangerous curve to the left',
'dangerous curve to the right',
'double curve',
'bumpy road',
'slippery road',
'road narrows on the right',
'road work',
'traffic signals',
'pedestrians',
'children crossing',
'bicycles crossing',
'beware of ice/snow',
'wild animals crossing',
'end of all speed and passing limits',
'turn right ahead',
'turn left ahead',
'ahead only',
'go straight or right',
'go straight or left',
'keep right',
'keep left',
'roundabout mandatory',
'end of no passing',
'end of no passing by vehicles over 3.5 metric tons'
]
colors = np.random.uniform(0, 255, size=(len(labels), 3))
# 加载测试图像
image = cv2.imread('test.jpg')
# 转换为 PyTorch 张量
image = torch.from_numpy(image / 255.0).float().permute(2, 0, 1)
# 将图像放入模型中进行预测
with torch.no_grad():
predictions = model([image])
# 获取预测结果
boxes = predictions[0]['boxes']
scores = predictions[0]['scores']
labels = predictions[0]['labels']
# 设置阈值,过滤掉低置信度的预测结果
threshold = 0.5
boxes = boxes[scores >= threshold]
labels = labels[scores >= threshold]
# 遍历每个交通标志区域,提取特征并进行分类
image = cv2.imread('test.jpg')
for box in boxes:
x1, y1, x2, y2 = box.cpu().numpy().astype(np.int32)
roi = image[y1:y2, x1:x2]
roi = cv2.resize(roi, (32, 32))
roi = torch.from_numpy(roi / 255.0).float().permute(2, 0, 1)
with torch.no_grad():
features = classifier(roi.unsqueeze(0))
probs = torch.softmax(features, dim=1)[0]
label = torch.argmax(probs)
color = colors[label]
cv2.rectangle(image, (x1, y1), (x2, y2), color, 2)
cv2.putText(image, labels[label], (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, color, 2)
# 显示图像
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()
```
注意,上述代码中的 `classifier.pth` 是训练好的交通标志分类模型的权重文件。
交通标志识别Resnet
ResNet是一种深度神经网络结构,常用于图像分类任务。在交通标志识别任务中,可以使用ResNet模型来对标志图像进行分类。ResNet模型的主要特点是使用残差连接(residual connection)来解决深度神经网络中的梯度消失问题,使得网络可以更深而不会导致性能下降。
具体地,可以使用已经预训练好的ResNet模型作为特征提取器,将输入的交通标志图像经过ResNet模型的卷积层和池化层得到一个固定长度的特征向量。然后,可以将这个特征向量输入到一个全连接层中进行分类。全连接层的输出是一个向量,每个元素代表一个类别的概率,可以使用softmax函数将其转换为概率分布。
在训练过程中,可以使用交叉熵损失函数来衡量模型预测结果与真实标签的差异,并使用反向传播算法来更新模型参数。在测试过程中,可以使用模型对新的交通标志图像进行分类,并输出概率分布。最终,可以选择概率最大的类别作为模型的预测结果。
需要注意的是,为了获得更好的分类性能,可以使用数据增强技术来扩充训练集。例如,可以对图像进行随机裁剪、旋转、缩放等操作,从而生成更多的训练样本。此外,还可以使用迁移学习技术,将已经在其他任务上预训练好的ResNet模型参数作为初始参数进行训练,可以加速模型的收敛并提高分类性能。
阅读全文
相关推荐
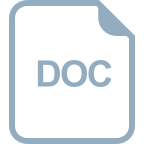
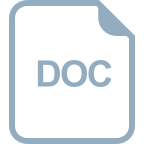
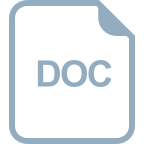
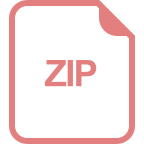
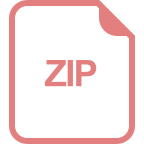
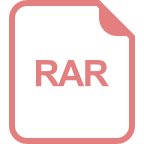
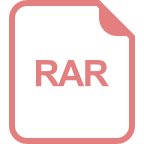
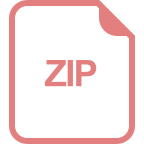
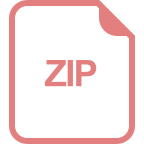
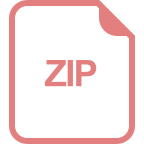
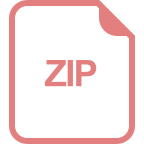
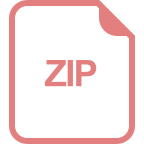
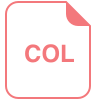
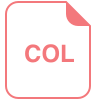
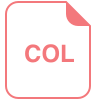
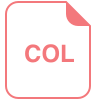
