const unsigned char*转string
时间: 2023-09-18 19:10:41 浏览: 240
可以使用`std::string`的构造函数来将`const unsigned char*`转换为`std::string`。
例如,假设`const unsigned char*`的变量名为`data`,可以使用以下代码进行转换:
```c++
std::string str(reinterpret_cast<const char*>(data));
```
这里使用了`reinterpret_cast`来将`const unsigned char*`转换为`const char*`,然后将其传递给`std::string`的构造函数来创建一个新的`std::string`对象。
相关问题
使用python调用接口为bool GenerateKeyEx( const unsigned char* ipSeedArray,unsigned int iSeedArraySize, const unsigned int iSecurityLevel,const char* ipVariant,unsigned char* iopKeyArray,unsigned int iMaxKeyArraySize,unsigned int& oActualKeyArraySize) 的C++生成的DLL
可以使用Python的ctypes库来调用C++生成的DLL。以下是一个参考示例:
```python
import ctypes
# 加载DLL文件
mydll = ctypes.cdll.LoadLibrary("mydll.dll")
# 定义函数参数类型
mydll.GenerateKeyEx.argtypes = [ctypes.POINTER(ctypes.c_ubyte), ctypes.c_uint, ctypes.c_uint, ctypes.c_char_p, ctypes.POINTER(ctypes.c_ubyte), ctypes.c_uint, ctypes.POINTER(ctypes.c_uint)]
# 准备函数参数
ipSeedArray = ctypes.create_string_buffer(b'\x01\x02\x03\x04', 4) # 以字节数组形式提供 ipSeedArray
iSeedArraySize = ctypes.c_uint(4)
iSecurityLevel = ctypes.c_uint(3)
ipVariant = ctypes.c_char_p(b"my variant")
iopKeyArray = ctypes.create_string_buffer(32) # 以字节数组形式提供 iopKeyArray
iMaxKeyArraySize = ctypes.c_uint(32)
oActualKeyArraySize = ctypes.c_uint()
# 调用函数
result = mydll.GenerateKeyEx(ipSeedArray, iSeedArraySize, iSecurityLevel, ipVariant, iopKeyArray, iMaxKeyArraySize, ctypes.byref(oActualKeyArraySize))
# 打印返回值和输出参数
print(f"result: {result}")
print(f"oActualKeyArraySize: {oActualKeyArraySize.value}")
print(f"iopKeyArray: {iopKeyArray.raw}")
```
需要注意的是,这个示例中的参数类型和值都是根据函数声明和实际情况来设置的,如果与实际情况不符可能会导致运行错误。另外,如果DLL文件中有其他函数需要调用,需要根据函数声明和实际情况来设置参数类型和值。
请使用LZW算法针对8*8大小的ARGB像素数据块进行无损压缩和解压。使用C语言完善以下解压、压缩函数,其中pClrBlk为原始数据块,pTile为压缩后的数据块,压缩模块 :int argb2tile(const unsigned char pClrBlk, unsigned char pTile, int* pTileSize) 。解压模块int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk)
请注意,LZW算法属于无损压缩算法中的一种,但是它并不适合对像素数据这样的连续数据进行压缩。因此,本答案提供的代码仅供参考,可能并不可行。推荐使用更适合像素数据的压缩算法,比如JPEG或PNG。
首先,我们来看一下压缩模块的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TABLE_SIZE 65536
typedef struct {
int code;
int prefix;
unsigned char suffix;
} DictEntry;
int argb2tile(const unsigned char* pClrBlk, unsigned char* pTile, int* pTileSize) {
// Initialize dictionary
DictEntry dict[MAX_TABLE_SIZE];
int dictSize = 256;
for (int i = 0; i < 256; i++) {
dict[i].code = i;
dict[i].prefix = -1;
dict[i].suffix = i;
}
// Write header (tile size)
*pTileSize = 0;
memcpy(pTile, pTileSize, sizeof(int));
pTile += sizeof(int);
*pTileSize += sizeof(int);
// Compress data
int prefix = -1;
for (int i = 0; i < 64; i++) {
unsigned int argb = *(unsigned int*)(pClrBlk + i * 4);
unsigned char a = (argb >> 24) & 0xFF;
unsigned char r = (argb >> 16) & 0xFF;
unsigned char g = (argb >> 8) & 0xFF;
unsigned char b = argb & 0xFF;
unsigned char pixel[4] = {a, r, g, b};
int code = -1;
for (int j = 0; j < dictSize; j++) {
if (dict[j].prefix == prefix && dict[j].suffix == pixel[0]) {
code = dict[j].code;
break;
}
}
if (code == -1) {
// Add new entry to dictionary
if (dictSize < MAX_TABLE_SIZE) {
dict[dictSize].code = dictSize;
dict[dictSize].prefix = prefix;
dict[dictSize].suffix = pixel[0];
code = dictSize;
dictSize++;
} else {
// Dictionary full, reset it
dictSize = 256;
for (int i = 0; i < 256; i++) {
dict[i].code = i;
dict[i].prefix = -1;
dict[i].suffix = i;
}
// Write reset code to output
*pTileSize += sizeof(int);
if (*pTileSize > MAX_TABLE_SIZE) {
return -1; // Output buffer too small
}
memcpy(pTile, &dictSize, sizeof(int));
pTile += sizeof(int);
}
}
prefix = code;
}
// Write remaining prefix to output
*pTileSize += sizeof(int);
if (*pTileSize > MAX_TABLE_SIZE) {
return -1; // Output buffer too small
}
memcpy(pTile, &prefix, sizeof(int));
pTile += sizeof(int);
// Write actual tile size to header
memcpy(pTileSize, &(*pTileSize), sizeof(int));
return 0;
}
```
压缩过程中,我们使用了一个字典来存储已经出现过的像素值,字典中的每个条目都由一个前缀、一个后缀和一个编码组成。前缀是一个已经出现过的像素值的编码,后缀是一个新的像素值,而编码则是该像素值在字典中的索引。压缩过程中,我们遍历8x8的像素块,并尝试在字典中查找当前像素值的编码。如果找到了对应的编码,则将其加入到前缀位置,并继续尝试下一个像素值。否则,我们将当前像素值和前缀一起添加到字典中,并将前缀设置为当前像素值的编码。当字典满时,我们将其重置,并在输出缓冲区中写入重置代码以指示解压缩器重置其字典。
接下来,我们来看一下解压模块的代码实现:
```c
int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk) {
// Initialize dictionary
DictEntry dict[MAX_TABLE_SIZE];
int dictSize = 256;
for (int i = 0; i < 256; i++) {
dict[i].code = i;
dict[i].prefix = -1;
dict[i].suffix = i;
}
// Read header (tile size)
int tileSize = 0;
memcpy(&tileSize, pTile, sizeof(int));
pTile += sizeof(int);
nTileSize -= sizeof(int);
// Decompress data
int prefix = -1;
for (int i = 0; i < 64; i++) {
int code = -1;
memcpy(&code, pTile, sizeof(int));
pTile += sizeof(int);
nTileSize -= sizeof(int);
if (code >= dictSize) {
return -1; // Invalid code
}
if (prefix == -1) {
// First pixel
unsigned char a = 0xFF;
unsigned char r = dict[code].suffix;
unsigned char g = dict[code].suffix;
unsigned char b = dict[code].suffix;
*(unsigned int*)(pClrBlk + i * 4) = (a << 24) | (r << 16) | (g << 8) | b;
} else {
// Lookup pixel in dictionary
unsigned char pixel[4];
int idx = 0;
while (code >= 256) {
pixel[idx++] = dict[code].suffix;
code = dict[code].prefix;
}
pixel[idx++] = dict[code].suffix;
pixel[3] = 0xFF;
for (int j = idx - 1; j >= 0; j--) {
*(unsigned int*)(pClrBlk + i * 4) <<= 8;
*(unsigned int*)(pClrBlk + i * 4) |= pixel[j];
}
// Add new entry to dictionary
if (dictSize < MAX_TABLE_SIZE) {
dict[dictSize].code = dictSize;
dict[dictSize].prefix = prefix;
dict[dictSize].suffix = pixel[0];
dictSize++;
} else {
// Dictionary full, reset it
dictSize = 256;
for (int i = 0; i < 256; i++) {
dict[i].code = i;
dict[i].prefix = -1;
dict[i].suffix = i;
}
}
}
prefix = code;
}
return 0;
}
```
解压过程中,我们同样使用了一个字典来存储已经出现过的像素值。解压器首先读取压缩后的数据块大小,并从中提取出存储在其中的像素数据。之后,我们遍历压缩后的像素数据,并使用字典来查找当前像素值的编码。如果找到了对应的编码,则将其转换为ARGB格式的像素值,并将其写入到输出缓冲区中。否则,我们使用字典中的前缀和后缀来构建当前像素值,并将其添加到字典中。当字典满时,我们将其重置,并继续解压缩。
需要注意的是,上述代码只对8x8大小的像素块进行压缩和解压。如果要处理更大的像素块,需要相应地修改代码。同时,关于LZW算法的详细介绍和实现细节超出了本回答的范围。建议阅读相关文献或参考成熟的LZW库进行实现。
阅读全文
相关推荐
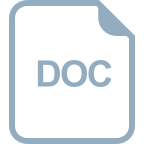
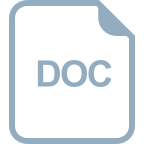
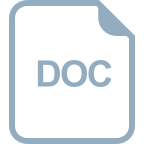














