#include <iostream> #include <vector> #include <algorithm> using namespace std; const int MAXN = 100; int n, m; int table[MAXN][MAXN], mask[MAXN], best_mask[MAXN]; int max_gain = 0; int calc_gain(int mask[]) { int gain = 0; for (int i = 0; i < n; i++) { int cnt = 0; for (int j = 0; j < m; j++) { if (mask[j] == 0 || table[i][j] == 0) { cnt++; } } if (cnt == m) { gain++; } } return gain; } void dfs(int cur, int cnt, int gain) { if (cur == m) { if (cnt > 0 && gain > max_gain) { max_gain = gain; for (int i = 0; i < m; i++) { best_mask[i] = mask[i]; } } return; } mask[cur] = 1; dfs(cur + 1, cnt + 1, gain + calc_gain(mask)); mask[cur] = 0; dfs(cur + 1, cnt, gain); } int main() { cin >> n >> m; for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { cin >> table[i][j]; } } dfs(0, 0, 0); cout << "The optimal attribute reduction result is:" << endl; cout << "Attribute index: "; for (int i = 0; i < m; i++) { if (best_mask[i] == 0) { cout << i << " "; } } cout << endl; return 0; }一句一句解释这段代码的意思
时间: 2024-02-14 16:23:54 浏览: 21
好的,我来一句一句解释这段代码的意思。
首先,这段代码使用了 C++ 语言标准库中的iostream、vector和algorithm头文件。
然后,定义了常量MAXN为100,表示二维数组的最大大小。
接下来定义了一些变量,包括n和m表示二维数组的行数和列数,table表示一个n*m的二维数组,mask和best_mask是大小为m的一维数组,用于记录属性是否被选择,max_gain表示当前最大的属性约简值。
calc_gain函数计算一个属性约简的值,具体实现是对于每一行,统计该行中未被选择的属性的数量,如果该行中所有的属性都未被选择,则该行可以被约简,gain值加1,最后返回总的gain值。
dfs函数是用于搜索属性组合的函数,递归地枚举每个属性是否被选择,记录当前的属性约简值和被选择的属性数量,当所有属性都被枚举后,计算出当前的属性约简值,如果该值大于之前的最大值,则更新最大值和最优选择的属性组合,最终得到最优的属性组合。
在主函数中,首先读入二维数组table,然后调用dfs函数搜索最优的属性组合,最后输出最优的属性组合。
总的来说,这段代码实现了一个属性约简的算法,通过枚举所有可能的属性组合,找到最优的属性约简结果。
相关问题
#include <iostream>#include <ctime>#include <cstdlib>using namespace std;const int MAXN = 500; // 需要排序的数组大小const int MAXV = 2000; // 随机数的最大值// 直接插入排序void insertSort(int a[], int
Sure, I can help you with that. The code you provided is written in C++ and it seems to be implementing the insertion sort algorithm. Insertion sort is a simple sorting algorithm that works by repeatedly inserting elements into a sorted portion of the array.
Here's the complete implementation of the insertion sort algorithm based on the code you provided:
```cpp
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
const int MAXN = 500; // Size of the array to be sorted
const int MAXV = 2000; // Maximum value for random numbers
// Function to generate random numbers
void generateRandomArray(int a[], int n) {
srand(time(0));
for (int i = 0; i < n; i++) {
a[i] = rand() % MAXV;
}
}
// Function to print the array
void printArray(int a[], int n) {
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
}
// Insertion sort implementation
void insertionSort(int a[], int n) {
for (int i = 1; i < n; i++) {
int key = a[i];
int j = i - 1;
while (j >= 0 && a[j] > key) {
a[j + 1] = a[j];
j--;
}
a[j + 1] = key;
}
}
int main() {
int arr[MAXN];
// Generate random array
generateRandomArray(arr, MAXN);
cout << "Original array: ";
printArray(arr, MAXN);
// Sort the array using insertion sort
insertionSort(arr, MAXN);
cout << "Sorted array: ";
printArray(arr, MAXN);
return 0;
}
```
This code generates a random array of size `MAXN` with values ranging from 0 to `MAXV`. It then sorts the array using the insertion sort algorithm and prints the original and sorted arrays.
Let me know if you have any further questions or if there's anything else I can assist you with!
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
相关推荐
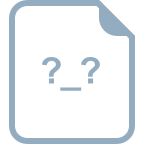













