实体字段integer 但是无法映射到excel的值 @ExcelProperty
时间: 2024-03-08 22:48:26 浏览: 104
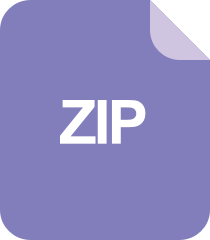
poi导出导入封装(使用注解建立实体与excel映射关系+反射设置实体属性值)
这个问题可能是由于导出时类型不匹配导致的。Excel中只能显示数字类型的数据,如果你的实体字段是 Integer 类型,但是在导出时没有通过转换成数字类型,就会导致无法映射到 Excel 的值。你可以尝试在对应的字段上添加注解 @NumberFormat,指定数字格式,或者在导出时将 Integer 类型转换成数字类型。例如:
```
@ExcelProperty(value = "实体字段", converter = IntegerConverter.class)
private Integer entityField;
```
其中,IntegerConverter 是一个自定义的转换器,实现了将 Integer 类型转换成数字类型的逻辑。
阅读全文
相关推荐
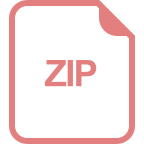
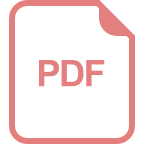
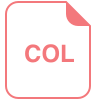
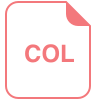


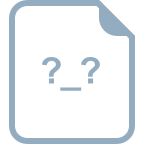
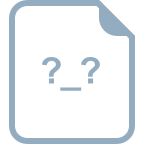
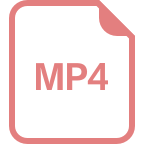
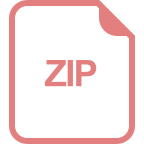