c语言题目题目8:字符文件处理 1)统计英文文本文件中单词的个数; 2)统计某一个特定单词出现的频度。
时间: 2023-10-23 22:34:29 浏览: 202
1) 统计英文文本文件中单词的个数可以按照以下步骤进行:
- 打开文本文件,读取文本内容。
- 将文本内容按照空格分割成单词。
- 统计单词的个数并输出。
以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
FILE *fp = fopen("file.txt", "r");
if (fp == NULL) {
printf("Failed to open file\n");
exit(1);
}
char word[50];
int word_count = 0;
while (fscanf(fp, "%s", word) != EOF) {
word_count++;
}
printf("Total words: %d\n", word_count);
fclose(fp);
return 0;
}
```
2) 统计某一个特定单词出现的频度可以按照以下步骤进行:
- 打开文本文件,读取文本内容。
- 将文本内容按照空格分割成单词。
- 遍历单词列表,统计特定单词出现的次数并输出。
以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
FILE *fp = fopen("file.txt", "r");
if (fp == NULL) {
printf("Failed to open file\n");
exit(1);
}
char word[50];
char target_word[50] = "apple";
int word_count = 0;
while (fscanf(fp, "%s", word) != EOF) {
if (strcmp(word, target_word) == 0) {
word_count++;
}
}
printf("Total occurrences of \"%s\": %d\n", target_word, word_count);
fclose(fp);
return 0;
}
```
阅读全文
相关推荐
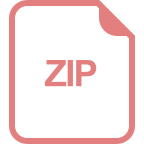

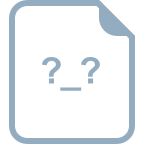
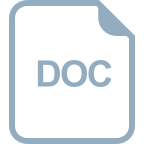
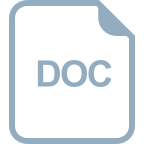
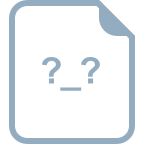
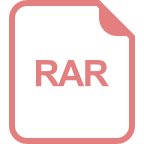
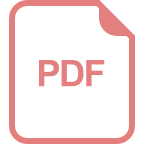
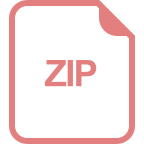
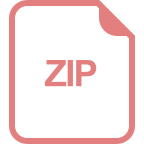