写个python代码 利用Faster R-CNN 模型如何给一张大图和一张小图,从大图中识别小图位置并返回小图在大图中的坐标
时间: 2024-03-04 13:49:37 浏览: 17
以下是一个简单的Python代码示例,演示如何使用Faster R-CNN模型在一张大图中定位一张小图的位置并返回其在大图中的坐标:
```python
import cv2
import torch
import numpy as np
from torchvision import transforms
# 加载预训练的 Faster R-CNN 模型
model = torch.load('faster_rcnn.pth')
model.eval()
# 定义图像预处理函数
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.485, 0.456, 0.406), (0.229, 0.224, 0.225))
])
# 加载大图和小图
image = cv2.imread('big_image.jpg')
small_image = cv2.imread('small_image.jpg')
# 将小图转换为张量并进行预处理
small_image_tensor = transform(small_image).unsqueeze(0)
# 在大图上进行滑动窗口操作,并对每个窗口进行目标检测
for i in range(0, image.shape[0] - small_image.shape[0], 10):
for j in range(0, image.shape[1] - small_image.shape[1], 10):
window = image[i:i+small_image.shape[0], j:j+small_image.shape[1]]
window_tensor = transform(window).unsqueeze(0)
with torch.no_grad():
outputs = model(window_tensor)
boxes = outputs['boxes']
labels = outputs['labels']
scores = outputs['scores']
# 判断是否检测到小图
if (labels == 1) and (scores > 0.9).any():
# 计算小图在大图中的坐标
x1, y1, x2, y2 = boxes[0].tolist()
x1 += j
x2 += j
y1 += i
y2 += i
print('小图在大图中的坐标:', x1, y1, x2, y2)
```
需要注意的是,上述代码仅是一个简单的示例,实际应用中需要根据具体情况进行调整和优化,以提高模型的检测精度和速度。
相关推荐
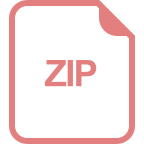
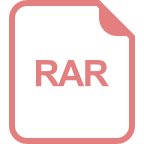
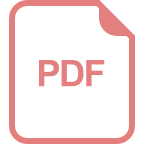
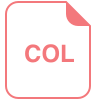
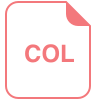
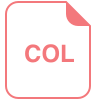
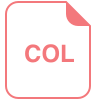
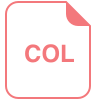









