controller URL传值
时间: 2023-09-12 20:13:21 浏览: 83
在控制器中,可以通过URL传递参数来获取特定的数据。这通常是通过使用路由来实现的。
例如,如果你有一个路由定义为:
```
Route::get('/user/{id}', 'UserController@show');
```
你可以在UserController中的show方法中获取id参数:
```
public function show($id)
{
$user = User::find($id);
return view('user.show', ['user' => $user]);
}
```
在这个例子中,通过URL传递的id参数被作为show方法的参数进行接收,并且使用它来查询用户数据。最后,将用户数据传递到视图中。
除了这种基本的用法之外,还有其他一些方法可以在控制器中使用URL传递参数。例如,你可以使用Request对象来获取URL参数:
```
public function show(Request $request)
{
$id = $request->input('id');
$user = User::find($id);
return view('user.show', ['user' => $user]);
}
```
在这个例子中,我们使用Request对象的input方法来获取id参数,并且使用它来查询用户数据。
相关问题
springboot controller传值
在Spring Boot中,你可以使用控制器(Controller)来处理HTTP请求并向客户端返回响应。在控制器中,你可以使用注解来定义方法以及传递参数。
要在控制器中传递值,你可以使用@RequestParam注解来获取HTTP请求中的参数,也可以使用@PathVariable注解来获取URL路径中的参数。此外,你还可以使用@RequestParam和@ModelAttribute注解来获取表单提交的参数。
下面是一个使用@RequestParam注解来传递值的例子:
```java
@RestController
public class MyController {
@GetMapping("/hello")
public String hello(@RequestParam("name") String name) {
return "Hello " + name;
}
}
```
在上面的例子中,当用户访问“/hello?name=world”时,控制器会返回“Hello world”的字符串。
另外,你还可以使用ModelAndView对象来在控制器中传递值,例如:
```java
@Controller
public class MyController {
@GetMapping("/hello")
public ModelAndView hello() {
ModelAndView mv = new ModelAndView();
mv.addObject("message", "Hello world!");
mv.setViewName("hello");
return mv;
}
}
```
在上面的例子中,控制器会返回名为“hello”的视图,并且将“Hello world!”传递给视图模板,你可以在模板中使用${message}来获取这个值。
springboot中controller如何向controller传值
在 Spring Boot 中,Controller 之间可以通过多种方式进行数据传递,下面介绍几种常用的方式:
1. 使用 RequestParam 或 PathVariable 传递参数
可以在请求的 URL 中通过 RequestParam 或 PathVariable 的方式传递参数,然后在接收参数的 Controller 方法中使用该参数。
示例代码如下:
```
@GetMapping("/user/{id}")
public String getUser(@PathVariable("id") Long id) {
// 处理业务逻辑
return "user";
}
@PostMapping("/user")
public String addUser(@RequestParam("name") String name, @RequestParam("age") Integer age) {
// 处理业务逻辑
return "redirect:/user/" + id;
}
```
2. 使用 Model 或 ModelMap 传递模型数据
可以在 Controller 方法中使用 Model 或 ModelMap 对象将模型数据传递给另一个 Controller 方法。
示例代码如下:
```
@GetMapping("/user/{id}")
public String getUser(@PathVariable("id") Long id, Model model) {
User user = userService.getUserById(id);
model.addAttribute("user", user);
return "user";
}
@PostMapping("/user")
public String addUser(User user, Model model) {
userService.addUser(user);
model.addAttribute("id", user.getId());
return "redirect:/user/{id}";
}
```
3. 使用 RedirectAttributes 传递重定向参数
可以在重定向时使用 RedirectAttributes 对象传递参数,然后在接收参数的 Controller 方法中使用该参数。
示例代码如下:
```
@PostMapping("/user")
public String addUser(User user, RedirectAttributes redirectAttributes) {
userService.addUser(user);
redirectAttributes.addAttribute("id", user.getId());
return "redirect:/user/{id}";
}
@GetMapping("/user/{id}")
public String getUser(@PathVariable("id") Long id, Model model) {
User user = userService.getUserById(id);
model.addAttribute("user", user);
return "user";
}
```
以上是常用的三种方式,具体使用哪种方式取决于具体业务需求。
阅读全文
相关推荐
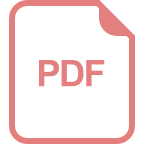
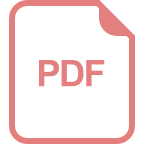
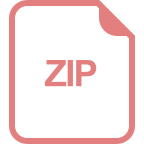













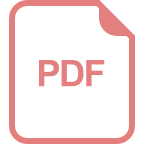