def build_model(max_features, maxlen): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model这个模型valid_chars = {x:idx+1 for idx, x in enumerate(set(''.join(X)))} max_features = len(valid_chars) + 1 maxlen = np.max([len(x) for x in X])生成的字典怎么实现get_config
时间: 2024-03-06 22:51:46 浏览: 112
您可以通过使用`get_config`函数来获取该字典。在这个模型中,您可以使用以下代码获取字典:
```
model = build_model(max_features, maxlen)
config = model.get_config()
```
`config`将是一个字典,其中包含有关模型的所有配置信息,包括嵌入层,LSTM层,Dropout层,密集层和激活函数。如果您想获取特定层的配置信息,您可以使用以下代码:
```
config = model.get_layer('layer_name').get_config()
```
其中 `layer_name` 是您要获取配置信息的层的名称。
相关问题
def build_model(max_features, maxlen): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model怎么model.build
这个函数中的模型是使用Keras的Sequential API构建的,而不是使用Functional API或Subclassing API。在这种情况下,我们不需要使用“model.build”方法来构建模型,因为它已经在“Sequential”类中自动完成了。
“Sequential”类是Keras中最简单的模型容器,它按顺序将各个网络层堆叠在一起,从而形成一个完整的神经网络模型。在这个函数中,我们首先使用“model.add”方法来添加一个嵌入层(Embedding)、LSTM层(LSTM)、Dropout层(Dropout)和一个密集层(Dense)到模型中,然后在最后添加一个sigmoid激活函数(Activation)来输出二元分类结果。
在这个函数中,我们已经定义好了模型的结构和参数,并使用“model.compile”方法来编译模型。这个方法接受损失函数(loss)、优化器(optimizer)和评估指标(metrics)等参数,然后构建出一个可训练的模型。最后,我们将这个模型返回给调用者。
def build_model(max_features=1, maxlen=65): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model def data(Domain): valid_chars = pickle.load(open('dictionary.plk','rb')) Domain = [[valid_chars[y] for y in x] for x in Domain] Domain = sequence.pad_sequences(Domain, maxlen=64) return Domain def run(Domain): Domains=data(Domain) model = build_model() model = model.load_weights('Mymodel.h5') predictions = model.predict(Domains) return predictions if __name__ == "__main__": print(run('baidu.com')) # Run with 1 to make it fast
在 `run` 函数中,使用 `model.load_weights` 方法加载模型权重时,不需要重新定义 `model`,直接使用之前定义的 `model` 即可。修改后的代码如下:
```python
def run(Domain):
Domains=data(Domain)
model = build_model()
model.load_weights('Mymodel.h5')
predictions = model.predict(Domains)
return predictions
```
此外,`run` 函数的参数需要传入一个列表或数组,因为 `data` 函数返回的是一个二维数组。修改后的代码如下:
```python
if __name__ == "__main__":
print(run(['baidu.com'])) # 注意需要传入一个列表或数组
```
另外,`build_model` 函数中的 `maxlen` 参数为 65,而在 `data` 函数中使用的是 64。需要保持一致。
阅读全文
相关推荐
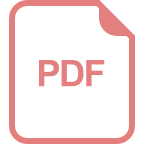
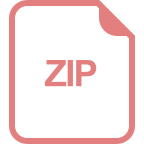
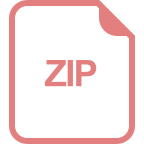






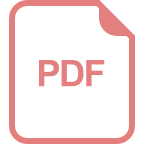
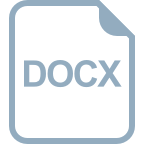
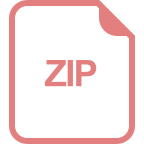
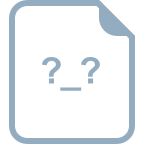
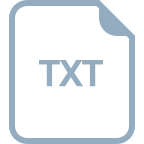
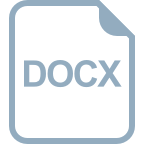