Traceback (most recent call last): File "D:\Programming\envs\env_pytorch\Lib\site-packages\IPython\core\interactiveshell.py", line 3508, in run_code exec(code_obj, self.user_global_ns, self.user_ns) File "<ipython-input-2-f56814941387>", line 1, in <module> runfile('D:\\Programming\\PycharmProjects\\P02_PIVmix\\Segment\\Image_filter.py', wdir='D:\\Programming\\PycharmProjects\\P02_PIVmix\\Segment') File "D:\Program Files\JetBrains\PyCharm Community Edition 2022.3.3\plugins\python-ce\helpers\pydev\_pydev_bundle\pydev_umd.py", line 198, in runfile pydev_imports.execfile(filename, global_vars, local_vars) # execute the script ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "D:\Program Files\JetBrains\PyCharm Community Edition 2022.3.3\plugins\python-ce\helpers\pydev\_pydev_imps\_pydev_execfile.py", line 18, in execfile exec(compile(contents+"\n", file, 'exec'), glob, loc) File "D:\Programming\PycharmProjects\P02_PIVmix\Segment\Image_filter.py", line 70, in <module> gamma_params, _ = curve_fit(gamma_func, new_bin_centers, new_hist, p0=p2) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "D:\Programming\envs\env_pytorch\Lib\site-packages\scipy\optimize\_minpack_py.py", line 859, in curve_fit res = leastsq(func, p0, Dfun=jac, full_output=1, **kwargs) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "D:\Programming\envs\env_pytorch\Lib\site-packages\scipy\optimize\_minpack_py.py", line 413, in leastsq shape, dtype = _check_func('leastsq', 'func', func, x0, args, n) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "D:\Programming\envs\env_pytorch\Lib\site-packages\scipy\optimize\_minpack_py.py", line 26, in _check_func res = atleast_1d(thefunc(*((x0[:numinputs],) + args))) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "D:\Programming\envs\env_pytorch\Lib\site-packages\scipy\optimize\_minpack_py.py", line 501, in func_wrapped return func(xdata, *params) - ydata ^^^^^^^^^^^^^^^^^^^^ File "D:\Programming\PycharmProjects\P02_PIVmix\Segment\Image_filter.py", line 63, in gamma_func assert isinstance(b, int) and b > 0, "b must be a positive integer" ^^^^^ AssertionError: b must be a positive integer
时间: 2023-07-21 20:46:05 浏览: 177
根据错误信息,可以看到程序出现了一个 AssertionError 异常,提示 "b must be a positive integer"。这个错误是由 gamma_func 函数中的 assert 语句触发的,说明输入参数 b 不满足要求。
根据代码,我们知道 gamma_func 函数要求输入参数 b 是正整数。因此,建议检查调用 gamma_func 函数时所传入的参数是否满足要求。如果不满足要求,可以进行相应的调整,确保输入参数 b 是正整数。
另外,如果需要捕获 AssertionError 异常并进行相应的处理,可以使用 try-except 语句来实现。例如:
```python
try:
gamma_params, _ = curve_fit(gamma_func, new_bin_centers, new_hist, p0=p2)
except AssertionError as e:
print("Error: ", e)
```
这里使用 try-except 语句捕获 AssertionError 异常,并输出相应的错误信息。
相关问题
Traceback (most recent call last): File D:\Programming\envs\env_pytorch\Lib\site-packages\spyder_kernels\py3compat.py:356 in compat_exec exec(code, globals, locals) File d:\programming\pycharmprojects\p02_pivmix\mix\main.py:287 plt.pcolormesh(pX, pY, M ,vmin=0, vmax=math.ceil(max(M)), cmap='inferno') ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
这个报错是因为 `vmax` 参数需要传入一个标量,但是你传入了一个数组 `math.ceil(max(M))`。
你可以将 `math.ceil(max(M))` 直接赋值给一个变量,然后将这个变量传给 `vmax` 参数。例如:
```python
import numpy as np
import math
import matplotlib.pyplot as plt
M = np.random.rand(10, 10) * 10
max_M = math.ceil(np.max(M))
pX, pY = np.meshgrid(np.arange(0, 10), np.arange(0, 10))
plt.pcolormesh(pX, pY, M ,vmin=0, vmax=max_M, cmap='inferno')
plt.colorbar()
plt.title('Displacement (pixels)')
plt.axis('scaled')
plt.savefig(result_path +'/2-D Velocity Field Displacement.png', dpi=300, bbox_inches='tight')
plt.show()
```
在这个例子中,我们首先使用NumPy库生成了一个10x10的随机二维数组M。然后,我们使用`np.max()`函数找到了数组M中的最大值,并使用`math.ceil()`函数对其进行向上取整,并将结果赋值给`max_M`变量。在调用`plt.pcolormesh()`函数时,我们将`max_M`作为`vmax`参数的值。最后,我们添加了一些其他的绘图设置,并将图像保存到指定的路径中。
Traceback (most recent call last): File "D:\anaconda\envs\PyTorch\lib\site-packages\chess\engine.py", line 1685, in play return await self.communicate(UciPlayCommand) File "D:\anaconda\envs\PyTorch\lib\site-packages\chess\engine.py", line 1094, in communicate return await command.result asyncio.exceptions.CancelledError During handling of the above exception, another exception occurred: Traceback (most recent call last): File "D:\anaconda\envs\PyTorch\lib\asyncio\tasks.py", line 492, in wait_for fut.result() asyncio.exceptions.CancelledError
这个错误信息看起来是在使用 Python 的 asyncio 库中出现的,似乎是因为一个协程被取消了,而在取消的同时又发生了另一个异常。具体原因可能需要查看更多的代码和上下文信息才能确定。你可以检查一下代码中使用 asyncio 的部分,看看是否有地方调用了 cancel() 方法或者手动取消了某个协程。另外,你也可以尝试加入更多的异常处理代码,以便更好地捕捉和处理异常。
阅读全文
相关推荐
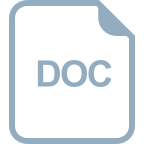
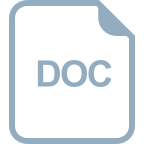
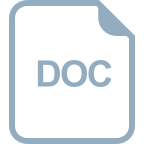













