用java对 https://www.3839.com/a/90818.htm 网址写一个爬取评论的爬虫
时间: 2023-11-21 18:04:22 浏览: 51
好的,下面是一个简单的爬取评论的示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class CommentCrawler {
public static void main(String[] args) throws Exception {
String urlString = "https://www.3839.com/a/90818.htm";
String html = getUrlHtml(urlString);
String commentUrl = getCommentUrl(html);
String commentHtml = getUrlHtml(commentUrl);
String commentText = getCommentText(commentHtml);
System.out.println(commentText);
}
/**
* 获取网页的HTML代码
*/
public static String getUrlHtml(String urlString) throws Exception {
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream(), "UTF-8"));
StringBuilder builder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
builder.append(line);
}
reader.close();
return builder.toString();
}
/**
* 获取评论的URL
*/
public static String getCommentUrl(String html) {
String regex = "<a href=\"(/ajax/.*?\\.html\\?id=\\d+)\">评论";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(html);
if (matcher.find()) {
return "https://www.3839.com" + matcher.group(1);
} else {
return "";
}
}
/**
* 获取评论的文本
*/
public static String getCommentText(String html) {
String regex = "<p class=\"text\">(.*?)</p>";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(html);
StringBuilder builder = new StringBuilder();
while (matcher.find()) {
String comment = matcher.group(1);
comment = comment.replaceAll("<.*?>", ""); // 去除HTML标签
comment = comment.replaceAll(" ", " "); // 替换空格
builder.append(comment).append("\n");
}
return builder.toString();
}
}
```
这段代码实现了以下功能:
1. 通过 `getUrlHtml()` 方法获取网页的 HTML 代码;
2. 通过 `getCommentUrl()` 方法从 HTML 代码中提取评论的URL;
3. 通过 `getUrlHtml()` 方法获取评论页面的 HTML 代码;
4. 通过 `getCommentText()` 方法从评论页面中提取评论文本,并且去掉 HTML 标签和替换空格。
注意:由于该网站使用了反爬机制,因此不能频繁地访问同一个页面,否则会被封 IP。建议设置适当的访问间隔,或者使用代理 IP。另外,使用爬虫需要遵守相关法律法规,不得用于非法用途。
相关推荐
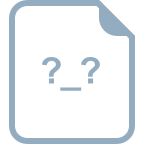
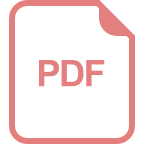














