void get_image(cv::VideoCapture &cap, Mat &image){ while(true){ cap >> image; this_thread::sleep_for(chrono::milliseconds (30)); } }
时间: 2024-03-05 13:50:06 浏览: 89
这段代码定义了一个名为 `get_image` 的函数,该函数使用了 OpenCV 库中的 `cv::VideoCapture` 类来从摄像头或者视频文件中读取帧,并将帧存储到 `cv::Mat` 对象中。该函数的参数包括一个 `cv::VideoCapture` 对象引用 `cap` 和一个 `cv::Mat` 对象引用 `image`。
函数的主要逻辑是通过 `cap >> image;` 从 `cap` 中读取一帧,并将其存储到 `image` 中。然后使用 `this_thread::sleep_for(chrono::milliseconds (30));` 函数使当前线程休眠 30 毫秒,以等待下一帧的到来。函数将一直循环执行,直到程序被手动中止。
该函数使用了 C++11 标准库中的线程库,需要包含头文件 `<thread>` 和 `<chrono>`。如果要在程序中使用该函数,需要先创建一个 `cv::VideoCapture` 对象,并将其作为参数传递给 `get_image` 函数,例如:
```c++
#include <opencv2/opencv.hpp>
#include <thread>
#include <chrono>
using namespace cv;
void get_image(VideoCapture& cap, Mat& image) {
while (true) {
cap >> image;
this_thread::sleep_for(chrono::milliseconds(30));
}
}
int main() {
VideoCapture cap(0); // 打开默认的摄像头
Mat image;
thread t(get_image, ref(cap), ref(image));
// 在这里可以对 image 进行处理,例如显示或保存到文件中
t.join();
return 0;
}
```
在主函数中,首先创建了一个默认的 `cv::VideoCapture` 对象 `cap`,用于打开默认摄像头。然后创建了一个 `cv::Mat` 对象 `image`,用于存储从摄像头中读取的帧。接着使用 `std::thread` 类创建了一个新线程,并将 `cap` 和 `image` 作为参数传递给 `get_image` 函数。在新线程中,函数将不断从 `cap` 中读取帧,并将其存储到 `image` 中。在主线程中,可以对 `image` 进行处理,例如显示或保存到文件中。最后使用 `t.join()` 等待新线程执行完毕。
阅读全文
相关推荐
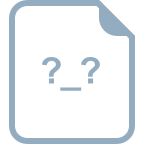
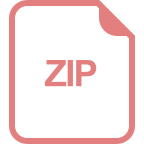
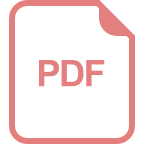
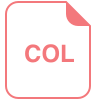
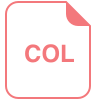
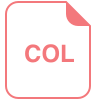
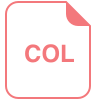
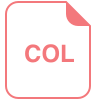
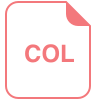
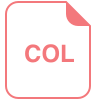
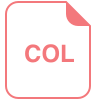
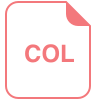
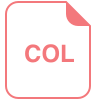
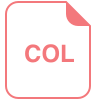
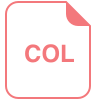
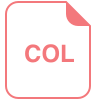
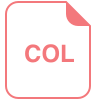
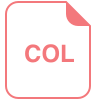