遗传算法代码示例,用python或C语言描述
时间: 2023-09-25 19:06:32 浏览: 86
### 回答1:
下面是一个使用 Python 的遗传算法的简单示例:
```python
import random
# 定义染色体长度
CHROMOSOME_LENGTH = 10
# 定义种群大小
POPULATION_SIZE = 20
# 定义交叉概率
CROSSOVER_RATE = 0.7
# 定义变异概率
MUTATION_RATE = 0.01
# 定义最大迭代次数
MAX_ITERATIONS = 100
# 生成初始种群
population = []
for i in range(POPULATION_SIZE):
chromosome = ''
for j in range(CHROMOSOME_LENGTH):
chromosome += str(random.randint(0, 1))
population.append(chromosome)
# 遗传算法主体
for i in range(MAX_ITERATIONS):
# 计算适应度
fitness = []
for j in range(POPULATION_SIZE):
count = 0
for k in range(CHROMOSOME_LENGTH):
if population[j][k] == '1':
count += 1
fitness.append(count)
# 选择父代
parents = []
for j in range(POPULATION_SIZE):
parents.append(population[fitness.index(max(fitness))])
fitness[fitness.index(max(fitness))] = -1
# 交叉
offspring = []
for j in range(int(POPULATION_SIZE/2)):
if random.random() < CROSSOVER_RATE:
crossover_point = random.randint(1, CHROMOSOME_LENGTH-1)
offspring.append(parents[j][:crossover_point] + parents[j+1][crossover_point:])
offspring.append(parents[j+1][:crossover_point] + parents[j][crossover_point:])
else:
offspring.append(parents[j])
offspring.append(parents[j+1])
# 变异
for j in range(POPULATION_SIZE):
if random.random() < MUTATION_RATE:
mutation_point = random.randint(0, CHROMOSOME_LENGTH-1)
offspring[j] = offspring[j][:mutation_point] + str(1-int(offspring[j][mutation_point])) + offspring[j][mutation_point+1:]
# 更新种群
population = offspring
# 输出最优解
print(population[fitness.index(max(fitness
### 回答2:
遗传算法是一种通过模拟生物进化过程来解决优化问题的算法。下面是一个用Python描述的遗传算法代码示例:
```python
import random
# 适应度函数(目标函数)
def fitness(solution):
# 根据问题的具体情况编写适应度函数,并返回适应度值
pass
# 生成初始种群
def generate_population(population_size, chromosome_length):
population = []
for _ in range(population_size):
chromosome = [random.randint(0, 1) for _ in range(chromosome_length)]
population.append(chromosome)
return population
# 交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异操作
def mutate(chromosome, mutation_rate):
for i in range(len(chromosome)):
if random.random() < mutation_rate:
chromosome[i] = 1 - chromosome[i]
return chromosome
# 选择操作
def selection(population, fitness_values):
total_fitness = sum(fitness_values)
probabilities = [fitness / total_fitness for fitness in fitness_values]
chosen_indices = random.choices(range(len(population)), probabilities, k=2)
return population[chosen_indices[0]], population[chosen_indices[1]]
# 遗传算法主函数
def genetic_algorithm(population_size, chromosome_length, mutation_rate, generations):
population = generate_population(population_size, chromosome_length)
for _ in range(generations):
fitness_values = [fitness(chromosome) for chromosome in population]
new_population = []
for _ in range(population_size // 2):
parent1, parent2 = selection(population, fitness_values)
child1, child2 = crossover(parent1, parent2)
child1 = mutate(child1, mutation_rate)
child2 = mutate(child2, mutation_rate)
new_population.extend([child1, child2])
population = new_population
return max(population, key=fitness)
# 调用遗传算法函数
result = genetic_algorithm(population_size=100, chromosome_length=10, mutation_rate=0.01, generations=50)
print(result)
```
上述代码实现了一个简单的遗传算法框架。根据具体问题的需求,需要编写适应度函数来评估个体的适应度。generate_population函数用于生成初始种群,crossover和mutate函数分别实现了交叉和变异操作,selection函数用于选择个体。最后调用genetic_algorithm函数传入相应的参数,即可运行遗传算法并得到优化结果。
注意:以上代码为简化示例,并没有完全实现遗传算法的所有细节,例如精英策略、种群更新策略等。根据具体问题的要求,可能需要在代码中进行相应的修改和优化。另外,遗传算法的效果还受到参数选择的影响,需要根据具体问题进行调整。
相关推荐
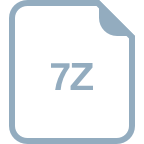














