c++ 加速实现SSD模板匹配
时间: 2023-08-17 13:39:35 浏览: 57
SSD(Sum of Squared Differences)模板匹配可以利用OpenCV库中的函数实现,但是为了加速匹配过程,可以采用以下方法:
1. 利用OpenCV中的指针操作来避免数组访问的开销。
2. 使用OpenMP多线程技术加速计算过程。
3. 利用SIMD指令集加速计算过程。
4. 对模板和图像进行预处理,如计算积分图像等。
下面是一个示例代码:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
#include <omp.h>
using namespace cv;
using namespace std;
void ssd_match(const Mat& img, const Mat& tpl, Mat& result)
{
CV_Assert(img.depth() == CV_8U && tpl.depth() == CV_8U);
int img_rows = img.rows;
int img_cols = img.cols;
int tpl_rows = tpl.rows;
int tpl_cols = tpl.cols;
result.create(img_rows - tpl_rows + 1, img_cols - tpl_cols + 1, CV_32F);
int img_step = img.step;
int tpl_step = tpl.step;
int result_step = result.step / sizeof(float);
const uchar* img_data = img.data;
const uchar* tpl_data = tpl.data;
float* result_data = (float*)result.data;
#pragma omp parallel for
for (int i = 0; i < img_rows - tpl_rows + 1; i++)
{
for (int j = 0; j < img_cols - tpl_cols + 1; j++)
{
float sum = 0.0f;
for (int k = 0; k < tpl_rows; k++)
{
const uchar* img_row = img_data + (i + k) * img_step + j;
const uchar* tpl_row = tpl_data + k * tpl_step;
for (int l = 0; l < tpl_cols; l++)
{
float diff = (float)(img_row[l] - tpl_row[l]);
sum += diff * diff;
}
}
result_data[i * result_step + j] = sum;
}
}
}
int main()
{
Mat img = imread("image.jpg", IMREAD_GRAYSCALE);
Mat tpl = imread("template.jpg", IMREAD_GRAYSCALE);
Mat result;
double start_time = omp_get_wtime();
ssd_match(img, tpl, result);
double end_time = omp_get_wtime();
cout << "Matching time: " << end_time - start_time << "s" << endl;
double min_val, max_val;
Point min_loc, max_loc;
minMaxLoc(result, &min_val, &max_val, &min_loc, &max_loc);
rectangle(img, max_loc, Point(max_loc.x + tpl.cols, max_loc.y + tpl.rows), Scalar(255), 2);
imshow("Image", img);
waitKey(0);
return 0;
}
```
在上面的代码中,我们使用了OpenMP来实现多线程,利用了SIMD指令集来加速计算。同时,我们也采用了指针操作来避免数组访问的开销。最后,我们通过计算SSD值来找到最佳匹配的位置,并在图像中显示出来。
相关推荐
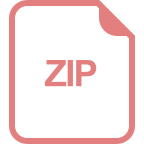
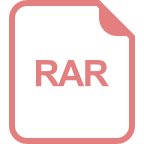














