write a c program that receives two integer parameters x and y , prints the value of x times y, then returns x.
时间: 2024-04-08 11:35:43 浏览: 20
Certainly! Here's a C program that receives two integer parameters `x` and `y`, prints the value of `x` times `y`, and then returns `x`:
```c
#include <stdio.h>
int multiplyAndReturnX(int x, int y) {
int result = x * y;
printf("The value of x times y is: %d\n", result);
return x;
}
int main() {
int x, y;
printf("Enter the value of x: ");
scanf("%d", &x);
printf("Enter the value of y: ");
scanf("%d", &y);
int returnValue = multiplyAndReturnX(x, y);
printf("The value of x is: %d\n", returnValue);
return 0;
}
```
In this program, the `multiplyAndReturnX` function takes two parameters `x` and `y`. It calculates the product of `x` and `y` and stores it in the `result` variable. Then, it prints the value of `x` times `y` using `printf`, and finally returns the value of `x`.
In the `main` function, it asks the user to enter the values of `x` and `y`. It calls the `multiplyAndReturnX` function with these values and stores the returned value in `returnValue`. Finally, it prints the value of `x` using `printf`.
相关推荐
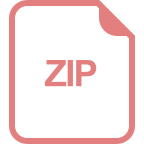
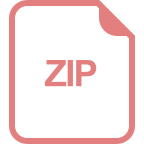
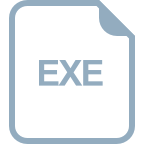














