c++的ll(1)分析法完整代码
时间: 2023-08-20 14:04:03 浏览: 45
以下是一个简单的C++ LL(1)分析法的完整代码示例,用于分析一个类C语言的简单语法:
```
#include <iostream>
#include <string>
#include <vector>
#include <map>
using namespace std;
// 定义文法符号类型
enum SymbolType { TERMINAL, NONTERMINAL };
// 定义文法符号结构体
struct Symbol {
SymbolType type; // 符号类型
string name; // 符号名称
};
// 定义产生式结构体
struct Production {
Symbol left; // 左部非终结符
vector<Symbol> right; // 右部符号序列
bool operator==(const Production& p) const {
return left.name == p.left.name && left.type == p.left.type && right == p.right;
}
};
// 定义预测分析表结构体
struct ParsingTable {
map<string, map<string, Production>> table; // 预测分析表
void addProduction(string nonterminal, string terminal, Production p) {
table[nonterminal][terminal] = p;
}
};
// 定义LL(1)文法
vector<Production> grammar = {
{ { NONTERMINAL, "Program" }, { { NONTERMINAL, "Stmt" }, { TERMINAL, ";" }, { NONTERMINAL, "Program" } } },
{ { NONTERMINAL, "Program" }, { { NONTERMINAL, "Stmt" } } },
{ { NONTERMINAL, "Stmt" }, { TERMINAL, "int" }, { TERMINAL, "id" } },
{ { NONTERMINAL, "Stmt" }, { { NONTERMINAL, "Expr" }, { TERMINAL, "=" }, { NONTERMINAL, "Expr" } } },
{ { NONTERMINAL, "Expr" }, { TERMINAL, "id" } },
{ { NONTERMINAL, "Expr" }, { TERMINAL, "num" } },
};
// 定义终结符集合和非终结符集合
vector<string> terminals = { "id", "num", ";", "=", "int" };
vector<string> nonterminals = { "Program", "Stmt", "Expr" };
// 构造LL(1)预测分析表
ParsingTable constructParsingTable() {
ParsingTable table;
for (auto& p : grammar) {
string A = p.left.name;
for (auto& a : terminals) {
if (a == p.right[0].name) {
table.addProduction(A, a, p);
}
}
if (p.right[0].type == NONTERMINAL) {
string B = p.right[0].name;
for (auto& a : terminals) {
if (p.right.size() > 1 && a == p.right[1].name) {
table.addProduction(B, a, p);
}
else {
for (auto& b : terminals) {
if (b != "epsilon" && table.table[B][b].left.name == B) {
table.addProduction(B, a, p);
}
}
}
}
}
}
return table;
}
// 解析输入文本串
bool parseInputString(string input, ParsingTable table) {
vector<Symbol> stack = { { NONTERMINAL, "Program" } };
int i = 0;
while (!stack.empty()) {
Symbol X = stack.back();
stack.pop_back();
if (X.type == TERMINAL) {
if (X.name == input.substr(i, X.name.size())) {
i += X.name.size();
}
else {
return false;
}
}
else {
Production p = table.table[X.name][input.substr(i, 1)];
if (p.right[0].name != "epsilon") {
for (int j = p.right.size() - 1; j >= 0; j--) {
stack.push_back(p.right[j]);
}
}
}
}
return i == input.size();
}
// 测试程序
int main() {
ParsingTable table = constructParsingTable();
string input;
cout << "请输入待分析的文本串:" << endl;
getline(cin, input);
if (parseInputString(input, table)) {
cout << "输入文本串符合文法规则!" << endl;
}
else {
cout << "输入文本串不符合文法规则!" << endl;
}
return 0;
}
```
以上代码仅作为示例,实际应用中需要根据具体文法和要分析的文本串进行相应的修改和调整。
相关推荐
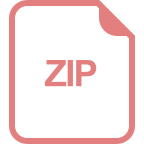
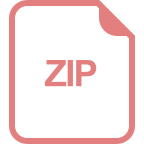














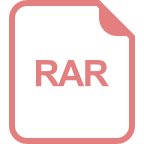