用C语言写一个解决01背包问题的程序,程序会输入一个文件,要求如下:The input txt file has 3 lines. The first line has only one integer, the capacity of the bag. The second line has a bunch of positive integers for the value of each item. Two integers are separated by a comma “,”. The third line is in the same format as the second for the weight of each item. - When the calculation is finished, print the optimal value and optimal solution(s) one for each line on the screen. (The optimal value is unique, but the optimal solution may not.) - Sort the items in the optimal solution(s) in the decreasing order of item indices. - The index of items starts from 1.
时间: 2023-06-26 19:08:16 浏览: 51
以下是解决01背包问题的C语言程序,可以实现读取输入txt文件,计算最优解并输出:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_ITEMS 1000
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s input_file\n", argv[0]);
return 1;
}
// 打开输入文件
FILE *input_file = fopen(argv[1], "r");
if (!input_file) {
printf("Failed to open input file.\n");
return 1;
}
// 读取背包容量
int capacity;
fscanf(input_file, "%d", &capacity);
// 读取物品价值和重量
int values[MAX_ITEMS], weights[MAX_ITEMS];
int item_count = 0;
while (!feof(input_file)) {
int value, weight;
fscanf(input_file, "%d,%d", &value, &weight);
if (value <= 0 || weight <= 0) {
break;
}
values[item_count] = value;
weights[item_count] = weight;
item_count++;
}
// 计算最优解
int dp[MAX_ITEMS + 1][capacity + 1];
for (int i = 0; i <= item_count; i++) {
for (int j = 0; j <= capacity; j++) {
if (i == 0 || j == 0) {
dp[i][j] = 0;
} else if (weights[i - 1] <= j) {
int include = values[i - 1] + dp[i - 1][j - weights[i - 1]];
int exclude = dp[i - 1][j];
dp[i][j] = include > exclude ? include : exclude;
} else {
dp[i][j] = dp[i - 1][j];
}
}
}
// 输出最优解
printf("%d\n", dp[item_count][capacity]);
for (int i = item_count, j = capacity; i > 0 && j > 0; i--) {
if (dp[i][j] != dp[i - 1][j]) {
printf("%d ", i);
j -= weights[i - 1];
}
}
printf("\n");
// 关闭输入文件
fclose(input_file);
return 0;
}
```
要使用该程序,需要将上述代码保存为一个C语言源文件(例如`knapsack.c`),然后使用编译器进行编译和链接,例如:
```
gcc -o knapsack knapsack.c
```
最后,运行程序时需要指定输入txt文件的路径作为参数,例如:
```
./knapsack input.txt
```
其中`input.txt`是包含背包容量、物品价值和重量的输入文件。
相关推荐
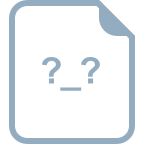














