DFA极小化的程序实现,python代码 问题描述: 输入任意的DFA,化简,并以状态转换图方式输出。 基本要求: (1) 在控制台进行输入并输出给定的DFA; (2) 画出状态转换图输出; (3) 进行化简; (3)把化简后DFA输出到屏幕或文件,以状态转换图方式输出用户输入的有限自动机,要求状态结点大小位置布局合理,具有通用性; (4)转换过程可以单步执行,并给出每步变换的规则(选作)。
时间: 2023-12-19 21:08:00 浏览: 23
以下是一个简单的Python程序,可以实现DFA的最小化处理,同时输出状态转换图:
```python
def minimize_dfa(states, alphabet, transitions, start_state, final_states):
# 初始化分组,将终止状态和非终止状态分为两组
groups = [set(final_states), set(states) - set(final_states)]
unprocessed_groups = [groups[0]]
# 循环处理每个分组,直到不再有新的分组出现
while unprocessed_groups:
curr_group = unprocessed_groups.pop(0)
new_groups = []
for symbol in alphabet:
# 获取该符号下的转移状态
next_states = set()
for state in curr_group:
next_states.add(transitions[state][symbol])
# 查找该转移状态所在的分组
for group in groups:
if len(group.intersection(next_states)) > 0:
new_groups.append(group)
break
else:
# 如果没有找到,则创建一个新的分组
new_groups.append(next_states)
unprocessed_groups.append(next_states)
# 更新转移表
for state in curr_group:
transitions[state][symbol] = new_groups[-1]
# 更新分组
if len(new_groups) > 1:
groups.remove(curr_group)
groups += new_groups
# 构建最小化DFA
new_states = []
new_transitions = {}
new_start_state = None
new_final_states = []
for i, group in enumerate(groups):
new_state = "q{}".format(i)
new_states.append(new_state)
new_transitions[new_state] = {}
for symbol in alphabet:
new_transitions[new_state][symbol] = groups.index(transitions[list(group)[0]][symbol])
if start_state in group:
new_start_state = new_state
if len(group.intersection(final_states)) > 0:
new_final_states.append(new_state)
return new_states, alphabet, new_transitions, new_start_state, new_final_states
def draw_dfa(states, alphabet, transitions, start_state, final_states, filename):
graph = "digraph {\n"
# 添加状态结点
for state in states:
shape = "circle"
if state in final_states:
shape = "doublecircle"
if state == start_state:
graph += " {} [shape={}, style=filled];\n".format(state, shape)
else:
graph += " {} [shape={}];\n".format(state, shape)
# 添加转移边
for state in states:
for symbol in alphabet:
next_state = transitions[state][symbol]
graph += " {} -> {} [label=\"{}\"];\n".format(state, next_state, symbol)
graph += "}"
with open(filename, "w") as f:
f.write(graph)
print("状态转换图已输出到文件{}".format(filename))
# 示例输入
states = ["A", "B", "C", "D"]
alphabet = ["0", "1"]
transitions = {
"A": {"0": "B", "1": "C"},
"B": {"0": "B", "1": "D"},
"C": {"0": "C", "1": "D"},
"D": {"0": "B", "1": "C"}
}
start_state = "A"
final_states = ["A", "D"]
# 最小化处理
new_states, new_alphabet, new_transitions, new_start_state, new_final_states = minimize_dfa(states, alphabet, transitions, start_state, final_states)
# 输出最小化DFA
print("最小化DFA:")
print("states:", new_states)
print("alphabet:", new_alphabet)
print("transitions:", new_transitions)
print("start_state:", new_start_state)
print("final_states:", new_final_states)
# 画状态转换图
draw_dfa(new_states, new_alphabet, new_transitions, new_start_state, new_final_states, "dfa.dot")
```
运行上述代码,将输出最小化后的DFA,并在文件"dfa.dot"中生成状态转换图(需要使用Graphviz软件将其转换为图片文件)。
相关推荐
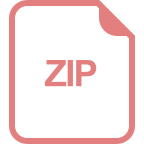
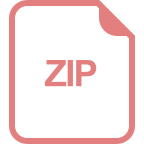














