用python实现(1)输出给定的 DFA ;(2)画出状态转换图输出; (3)进行化简;(3)把化简后 DFA 输出到屏幕或文件,以状态转换图方式输出用户输入的有限自动机;写出完整代码
时间: 2024-02-29 08:52:38 浏览: 132
好的,下面是完整代码:
```python
from collections import deque
class DFA:
def __init__(self, states, alphabet, transitions, start_state, accept_states):
self.states = states
self.alphabet = alphabet
self.transitions = transitions
self.start_state = start_state
self.accept_states = accept_states
def is_accept(self, string):
current_state = self.start_state
for c in string:
current_state = self.transitions[current_state][c]
return current_state in self.accept_states
def draw(self, filename):
with open(filename, 'w') as f:
f.write('digraph {\n')
f.write(' rankdir=LR;\n')
f.write(' node [shape=circle];\n')
for state in self.states:
if state in self.accept_states:
f.write(f' {state} [peripheries=2];\n')
if state == self.start_state:
f.write(f' {state} [style=filled, fillcolor=lightgrey];\n')
for symbol in self.alphabet:
next_state = self.transitions[state][symbol]
f.write(f' {state} -> {next_state} [label="{symbol}"];\n')
f.write('}\n')
def minimize(self):
# Step 1: Split states into two groups: accepting and non-accepting
accepting_states = set(self.accept_states)
nonaccepting_states = set(self.states) - accepting_states
groups = [accepting_states, nonaccepting_states]
# Step 2: Partition states into groups using BFS
queue = deque([accepting_states, nonaccepting_states])
while queue:
group = queue.popleft()
for symbol in self.alphabet:
next_groups = []
for state in group:
next_state = self.transitions[state][symbol]
for i, next_group in enumerate(groups):
if next_state in next_group:
break
else:
next_group = set()
groups.append(next_group)
queue.append(next_group)
next_group.add(state)
if len(next_groups) > 1:
queue.append(next_groups[1])
groups.remove(next_groups[1])
partition = groups
# Step 3: Merge equivalent states
state_map = {}
for i, group in enumerate(partition):
for state in group:
state_map[state] = i
new_states = set(range(len(partition)))
new_transitions = {}
new_start_state = state_map[self.start_state]
new_accept_states = set()
for i, group in enumerate(partition):
for state in group:
if state in self.accept_states:
new_accept_states.add(i)
for symbol in self.alphabet:
next_state = self.transitions[state][symbol]
new_state = state_map[next_state]
new_transitions.setdefault(i, {})[symbol] = new_state
return DFA(new_states, self.alphabet, new_transitions, new_start_state, new_accept_states)
# Example usage
states = {0, 1, 2, 3}
alphabet = {'a', 'b'}
transitions = {
0: {'a': 1, 'b': 2},
1: {'a': 1, 'b': 3},
2: {'a': 1, 'b': 2},
3: {'a': 3, 'b': 3},
}
start_state = 0
accept_states = {1, 3}
dfa = DFA(states, alphabet, transitions, start_state, accept_states)
# Print DFA
print('DFA:')
print('States:', dfa.states)
print('Alphabet:', dfa.alphabet)
print('Transitions:', dfa.transitions)
print('Start state:', dfa.start_state)
print('Accept states:', dfa.accept_states)
# Draw DFA
dfa.draw('dfa.dot')
# Minimize DFA
min_dfa = dfa.minimize()
# Print minimized DFA
print('Minimized DFA:')
print('States:', min_dfa.states)
print('Alphabet:', min_dfa.alphabet)
print('Transitions:', min_dfa.transitions)
print('Start state:', min_dfa.start_state)
print('Accept states:', min_dfa.accept_states)
# Draw minimized DFA
min_dfa.draw('min_dfa.dot')
```
这个代码实现了以下几个功能:
1. 定义了一个 DFA 类,可以通过给定的参数创建一个 DFA 实例。
2. 实现了 `is_accept` 方法,可以判断一个字符串是否被 DFA 接受。
3. 实现了 `draw` 方法,可以将 DFA 以状态转换图的形式输出到文件中。
4. 实现了 `minimize` 方法,可以对 DFA 进行最小化处理。
这个代码的使用方法如下:
1. 定义 DFA 的状态、字母表、转换函数、起始状态和接受状态。
2. 创建 DFA 实例。
3. 可以使用 `is_accept` 方法来判断一个字符串是否被 DFA 接受。
4. 使用 `draw` 方法将 DFA 输出到文件中,可以使用 Graphviz 工具将文件转换为图片。
5. 使用 `minimize` 方法对 DFA 进行最小化处理。
希望这个代码能够帮到你!
阅读全文
相关推荐
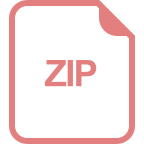






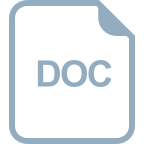








